3 回答
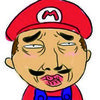
TA贡献1851条经验 获得超5个赞
您可以将问题分为三部分:
首先创建两个列表,一个包含按遇到的顺序排列的偶数,另一个包含奇数:
private static List<List<Integer>> createOddityLists(int... numbers) {
List<Integer> numsList = Arrays.stream(numbers).boxed().collect(Collectors.toList());
List<List<Integer>> numsByOddity = new ArrayList<List<Integer>>();
numsByOddity.add(new ArrayList<>()); // List of odd numbers
numsByOddity.add(new ArrayList<>()); // List of even numbers
numsList.forEach(num -> numsByOddity.get(num % 2).add(num));
return numsByOddity;
}
用零 ( s) 填充两个列表中较短的一个,0使其与另一个列表的长度相等:
private static void padShorterList(List<List<Integer>> numsByOddity) {
int sizeDiff = numsByOddity.get(0).size() - numsByOddity.get(1).size();
int listIndexToBePadded = sizeDiff < 0 ? 0 : 1;
List<Integer> padding = Collections.nCopies(Math.abs(sizeDiff), 0);
numsByOddity.get(listIndexToBePadded).addAll(padding);
}
最后连接两个列表:
private static List<Integer> joinLists(List<List<Integer>> numsByOddity) {
List<Integer> resultList = new ArrayList<>(numsByOddity.get(1));
for (int idx = 0; idx < numsByOddity.get(0).size(); idx++)
resultList.add(idx * 2, numsByOddity.get(0).get(idx));
return resultList;
}
以下是完整的工作示例:
public class ArrayRearrangement {
public static void main(String[] args) {
// int[] result = rearrange(1, 2, 3, 4);
int[] result = rearrange(1, 1, 1, 4);
System.out.println(Arrays.stream(result).boxed().collect(Collectors.toList()));
}
private static int[] rearrange(int... numbers) {
List<List<Integer>> numsByOddity = createOddityLists(numbers);
padShorterList(numsByOddity);
return joinLists(numsByOddity).stream().mapToInt(i->i).toArray();
}
private static List<List<Integer>> createOddityLists(int... numbers) {
List<Integer> numsList = Arrays.stream(numbers).boxed().collect(Collectors.toList());
List<List<Integer>> numsByOddity = new ArrayList<List<Integer>>();
numsByOddity.add(new ArrayList<>()); // List of odd numbers
numsByOddity.add(new ArrayList<>()); // List of even numbers
numsList.forEach(num -> numsByOddity.get(num % 2).add(num));
return numsByOddity;
}
private static void padShorterList(List<List<Integer>> numsByOddity) {
int sizeDiff = numsByOddity.get(0).size() - numsByOddity.get(1).size();
int listIndexToBePadded = sizeDiff < 0 ? 0 : 1;
List<Integer> padding = Collections.nCopies(Math.abs(sizeDiff), 0);
numsByOddity.get(listIndexToBePadded).addAll(padding);
}
private static List<Integer> joinLists(List<List<Integer>> numsByOddity) {
List<Integer> resultList = new ArrayList<>(numsByOddity.get(1));
for (int idx = 0; idx < numsByOddity.get(0).size(); idx++)
resultList.add(idx * 2, numsByOddity.get(0).get(idx));
return resultList;
}
}
希望这可以帮助。
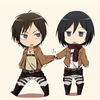
TA贡献1821条经验 获得超4个赞
根据索引对元素进行排序,即如果元素是偶数,则它必须位于偶数位置,反之亦然
int sortArrayByEvenOddIndex(int arr[]) {
int n = arr.length;
int res[] = new int[n];
int odd = 1;
int even = 0;
for (int i = 0; i < n; i++) {
if (arr[i] % 2 == 0) {
res[even] = arr[i];
even += 2;
} else {
res[odd] = arr[i];
odd += 2;
}
}
return res;
}
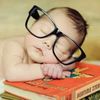
TA贡献1757条经验 获得超7个赞
使用数组我们可以做到这一点。代码需要优化。
public static int[] arrangeInEvenOddOrder(int[] arr)
{
// Create odd and even arrays
int[] oddArr = new int[arr.length];
int[] evenArr = new int[arr.length];
int oCount = 0, eCount = 0;
// populate arrays even and odd
for (int i = 0; i < arr.length; i++) {
if (arr[i] % 2 == 0)
evenArr[eCount++] = arr[i];
else
oddArr[oCount++] = arr[i];
}
int[] resArr = new int[oCount >= eCount?
2*oCount : 2*eCount-1];
// populate elements upto min of the
// two arrays
for (int i =0; i < (oCount <= eCount?
2*oCount : 2*eCount ); i++ )
{
if( i%2 == 0)
resArr[i] = evenArr[i/2];
else
resArr[i] = oddArr[i/2];
}
// populate rest of elements of max array
// and add zeroes
if (eCount > oCount)
{
for (int i=2*oCount,j=0;i<2*eCount-1; i++)
{
if (i%2 == 0)
{
resArr[i] = evenArr[oCount+j];
j++;
}
else
resArr[i] = 0;
}
}
else if (eCount < oCount)
{
for (int i=2*eCount,j=0;i<2*oCount; i++)
{
if ( i%2 != 0)
{
resArr[i] = oddArr[eCount+j];
j++;
}
else
resArr[i] = 0;
}
}
return resArr;
}
添加回答
举报