2 回答
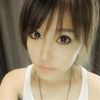
TA贡献1951条经验 获得超3个赞
正如已经评论过的,这与多线程无关。由于数字是随机的,因此它们也有可能相同。
如果你想要 2 个不相同的随机数,你可以这样做:
//Create List of integers
List<Integer> numberPool = new ArrayList<>();
//Initialize list with numbers from 1 to 1000
for(int num = 1; num <= 1000 ; num++) {
numberPool.add(num);
}
//Randomly shuffle list
Collections.shuffle(numberPool);
//Get first number
System.out.println(numberPool.get(0));
//Get second number
System.out.println(numberPool.get(1));
如果你想以多线程方式访问 numberPool,你可以这样做:
public class NumGeneratorThreadSafe{
List<Integer> numberPool = new ArrayList<>();
private int counter = 0;
public NumGeneratorThreadSafe() {
for(int i = 1; i <= 1000 ; i++) {
this.numberPool.add(i);
}
Collections.shuffle(this.numberPool);
}
public synchronized Integer getRandomNumber() {
return this.numberPool.get(this.counter++);
}
public synchronized void resetCounter() {
this.counter = 0;
}
}
希望这可以帮助。
编辑: 为了在线程中使用该类:
public class MultiThreadingRandom {
public static void main(String[] args) throws InterruptedException {
ExecutorService executorService = Executors.newFixedThreadPool(2);
NumGeneratorThreadSafe numGenerator = new NumGeneratorThreadSafe();
GeneRan r1 = new GeneRan(numGenerator);
GeneRan r2 = new GeneRan(numGenerator);
executorService.submit(r1);
executorService.submit(r2);
executorService.shutdown();
}
}
class GeneRan implements Runnable{
private NumGeneratorThreadSafe numGenerator;
public GeneRan(NumGeneratorThreadSafe numGenerator) {
this.numGenerator = numGenerator;
}
@Override
public void run() {
int randInt = this.numGenerator.getRandomNumber();
System.out.println(randInt);
}
}
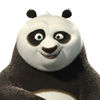
TA贡献2012条经验 获得超12个赞
这个怎么样
import java.util.Random;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class MultiThreadingRandom {
public static void main(String[] args) throws InterruptedException, ExecutionException {
ExecutorService executorService = Executors.newFixedThreadPool(2);
Generun r1 = new Generun();
executorService.submit(r1);
Thread.sleep(100);
executorService.submit(r1);
executorService.shutdown();
}
}
class Generun implements Runnable {
int nextInt = -1;
int bound = 1000;
@Override
public void run() {
int temp = nextInt;
nextInt = new Random().nextInt(bound);
if (temp == nextInt) {
do {
nextInt = new Random().nextInt(bound);
} while (temp == nextInt);
}
System.out.println(nextInt);
}
}
添加回答
举报