2 回答
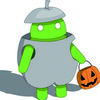
TA贡献1982条经验 获得超2个赞
只需使用队列创建一个简单的前序遍历即可。
from queue import Queue
def preorder(root):
ans = []
q = Queue()
q.put(root)
while not q.empty():
temp = []
n = q.qsize()
while n:
x = q.get()
n-=1
temp.append(x.data)
if x.left:
q.put(x.left)
if x.right:
q.put(x.right)
ans.append(temp)
print(ans[::-1]) # prints [[1], [2], [3, 5], [4]] for your example
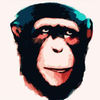
TA贡献1790条经验 获得超9个赞
我(终于)解决了这个问题,在尝试了 5 个小时的不同解决方案后,我回到了这个问题并纠正了它。这是正确的代码:
import bst
def preorder(root, level, dict):
# base case: empty tree
if root is None:
return
# insert current node and its level into the dict
dict.setdefault(level, []).append(root.data)
# recur for left and right subtree by increasing level by 1
if root.left is not None:
preorder(root.left, level + 1, dict)
if root.right is not None:
preorder(root.right , level + 1, dict)
# Recursive function to do reverse level order traversal of given binary tree
def tree_levels(tree):
list = []
# create an empty dict to store nodes between given levels
dict = {}
# traverse the tree and insert its nodes into the dict
# corresponding to the their level
preorder(tree, 0, dict)
# iterate through the dict in reverse order and
# print all nodes present in very level
for i in range(len(dict)-1,-1,-1):
list += dict[i]
return list
添加回答
举报