2 回答
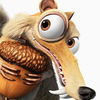
TA贡献1829条经验 获得超13个赞
属性是一种方法,但如果您想避免使用反射,您可以实现一个包含您需要的所有内容的类,类似于:
public enum UnitType
{
Second,
Microsecond,
Millisecond,
Minute,
Hour,
Day,
Week
}
public class Unit
{
public string Name { get; private set; }
public string Symbol { get; private set; }
public double Factor { get; private set; }
public Unit Base { get; private set; }
public Unit(UnitType unit, bool isBase = false)
{
Name = GetUnitName(unit);
Symbol = GetUnitSymbol(unit);
Factor = GetUnitFactor(unit);
if (!isBase)
Base = GetUnitBase(unit);
}
private string GetUnitName(UnitType unit)
{
switch (unit)
{
case UnitType.Second:
return "second";
case UnitType.Microsecond:
return "microsecond";
case UnitType.Millisecond:
return "millisecond";
case UnitType.Minute:
return "minute";
case UnitType.Hour:
return "hour";
case UnitType.Day:
return "day";
case UnitType.Week:
return "week";
default:
return null;
}
}
private string GetUnitSymbol(UnitType unit)
{
switch (unit)
{
case UnitType.Second:
return "s";
case UnitType.Microsecond:
return "μs";
case UnitType.Millisecond:
return "ms";
case UnitType.Minute:
return "min";
case UnitType.Hour:
return "h";
case UnitType.Day:
return "d";
case UnitType.Week:
return "w";
default:
return null;
}
}
private double GetUnitFactor(UnitType unit)
{
switch (unit)
{
case UnitType.Second:
return 1;
case UnitType.Microsecond:
return 0.000001;
case UnitType.Millisecond:
return 0.001;
case UnitType.Minute:
return 60.0;
case UnitType.Hour:
return 3600.0;
case UnitType.Day:
return 24.0;
case UnitType.Week:
return 7;
default:
return 0;
}
}
private Unit GetUnitBase(UnitType unit)
{
switch (unit)
{
case UnitType.Microsecond:
return new Unit(UnitType.Second, true);
case UnitType.Millisecond:
return new Unit(UnitType.Second, true);
case UnitType.Minute:
return new Unit(UnitType.Second, true);
case UnitType.Hour:
return new Unit(UnitType.Minute, true);
case UnitType.Day:
return new Unit(UnitType.Hour, true);
case UnitType.Week:
return new Unit(UnitType.Day, true);
default:
return null;
}
}
}
用法 :
// initiate a new Unit instance.
var unit = new Unit(UnitType.Week);
// Get values
var name = unit.Name;
var symbol = unit.Symbol;
var factor = unit.Factor;
// In case if some units doesn't have base
if (unit.Base != null)
{
var baseName = unit.Base.Name;
var baseSymbol = unit.Base.Symbol;
var baseFactor = unit.Base.Factor;
}
这只是一个简单的示例,尚未经过充分测试,只是向您展示另一种可以实现的方法。
您还可以使用隐式运算符来获取值 例子 :
public class Unit
{
......
public static implicit operator double(Unit v) => v.Factor;
public static implicit operator string(Unit v) => v.Symbol;
}
隐式获取值:
var symbol = (string) unit; // will return the v.Symbol
var factor = (double) unit; // will return the v.Factor
此外,我没有展示UnitTypes.Time,因为没有太多关于它的代码,但我认为示例和想法足以为您的想法提供所需的推动力。
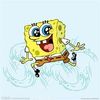
TA贡献1816条经验 获得超4个赞
我不确定你到底想做什么,但我认为属性将是一个不错的选择。您可以像这样在每个单元枚举字段上设置属性,并在需要时通过反射检索它们。
[AttributeUsage(AttributeTargets.Field, Inherited = false, AllowMultiple = false)]
sealed class UnitTypeAttribute : Attribute
{
public UnitType UnitType{ get; set; }
public UnitAttribute(UnitType unitT)
{
UnitType= unitT;
}
}
enum Unit
{
[UnitType(UnitTypes.Time)]
Second,
[UnitType(UnitTypes.Time)]
MicroSecond,
[UnitType(UnitTypes.Time)]
Hour
}
然后,当你想检索它时,使用此方法(可以通用)
public static UnitType GetUnitTypeAttribute(Unit unit)
{
var memberInfo = typeof(Unit).GetMember(unit.ToString());
var result = memberInfo[0].GetCustomAttributes<UnitTypeAttribute>(false)
return ((UnitType)result).UnitType;
}
- 2 回答
- 0 关注
- 167 浏览
添加回答
举报