3 回答
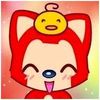
TA贡献1864条经验 获得超6个赞
为了移动数组中的数字,以下 for 循环可用于移动数组中的值。
// prerequisite: array is already filled with values
for(int i = 0; i < numArray.length; i++) {
arr[i] += shiftNum;
if (numArray[i] > 30) {
numArray[i] -= 30;
} else if (numArray[i] <= 0) {
numArray[i] += 30;
}
}
根据您的代码,创建的数组将包含 1 - 30 之间的值,包括 1 和 30。如果您希望代码包含 0 - 29 之间的值,请将 numArray[i] > 30 更改为 numArray[i] >= 30 并将 numArray[i] <= 0 更改为 numArray[i] < 0。
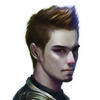
TA贡献2003条经验 获得超2个赞
使用 Java 的便捷方法。大多数人仍然想编写 for 循环。基本上,您需要保存通过转变覆盖的元素。然后将那些保存的放回数组中。System.arraycopy 很好,因为它可以处理数组中移动元素的一些令人讨厌的部分。
void shift(int shiftBy, int... array) {
int[] holdInts = Arrays.copyOf(array, shiftBy);
System.arraycopy(array, shiftBy, array, 0, array.length - shiftBy);
System.arraycopy(holdInts, 0, array, array.length - shiftBy, holdInts.length);
}
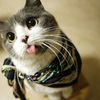
TA贡献1811条经验 获得超6个赞
这是为您提供的快速解决方案。请检查以下代码。
输入 :
输入移位/旋转:4
输出 :
旋转给定数组 [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30]
旋转后 [27, 28, 29, 30, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 , 21, 22, 23, 24, 25, 26]
public static void main(String[] args) {
RotationDemo rd = new RotationDemo();
int[] input = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30};
int k = 0;
Scanner scan = new Scanner (System.in);
try{
System.out.println("\nEnter the shift/rotation:");
int shiftNum = scan.nextInt();
if(shiftNum < 30) {
k = shiftNum;
System.out.println("Rotate given array " + Arrays.toString(input));
int[] rotatedArray = rd.rotateRight(input, input.length, k);
System.out.println("After Rotate " +
Arrays.toString(rotatedArray));
} else {
System.out.println("Shift number should be less than 30");
}
} catch(Exception ex){
} finally {
scan.close();
}
}
public int[] rotateRight(int[] input, int length, int numOfRotations) {
for (int i = 0; i < numOfRotations; i++) {
int temp = input[length - 1];
for (int j = length - 1; j > 0; j--) {
input[j] = input[j - 1];
}
input[0] = temp;
}
return input;
}
希望这个例子能起作用。
添加回答
举报