4 回答
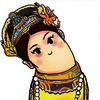
TA贡献1842条经验 获得超12个赞
如果是包含对象键的字符串,则它将units[n][m]起作用。m
example units[0]["code"]将返回第一个对象的代码值。
填充表可以通过在字符串中动态生成 html 并将table.innerHTML其设置为表来完成。
为此,我们使用以下方法迭代键for (let key in object)
对于列名称
let tableString="<tr>"
for(let column in units[0]){
tableString+=`<th>${column}</th>`
}
上面的代码将生成一个像这样的字符串
<tr>
<th>code</th>
<th>title</th>
<th>offering</th>
</tr>
对于列数据
tableString+="</tr>"
units.forEach(element => {
tableString+="<tr>"
for(let prop in element){
tableString+=`<td>${element[prop]}</td>`
}
tableString+="</tr>"
});
上面将生成这样的字符串,并且这将重复直到数组末尾
<tr>
<td>COMP2110</td>
<td>Web Technology</td>
<td>S1</td>
</tr>
<tr>...
</tr>
<tr>...
</tr>
最后
document.querySelector('#tb').innerHTML=tableString;
let units = [
{
'code': 'COMP2110',
'title': 'Web Technology',
'offering': 'S1'
},
{
'code': 'COMP2010',
'title': 'Algorithms and Data Structures',
'offering': 'S1'
},
{
'code': 'COMP2150',
'title': 'Game Design',
'offering': 'S1'
},
{
'code': 'COMP2320',
'title': 'Offensive Security',
'offering': 'S1'
},
{
'code': 'COMP2200',
'title': 'Data Science',
'offering': 'S2'
},
{
'code': 'COMP2250',
'title': 'Data Communications',
'offering': 'S2'
},
{
'code': 'COMP2300',
'title': 'Applied Cryptography',
'offering': 'S2'
},
{
'code': 'COMP2000',
'title': 'Object-Oriented Programming Practices',
'offering': 'S2'
},
{
'code': 'COMP2050',
'title': 'Software Engineering',
'offering': 'S2'
},
{
'code': 'COMP2100',
'title': 'Systems Programming',
'offering': 'S2'
}
]
let tableString="<tr>"
for(let column in units[0]){
tableString+=`<th>${column}</th>`
}
tableString+="</tr>"
units.forEach(element => {
tableString+="<tr>"
for(let prop in element){
tableString+=`<td>${element[prop]}</td>`
}
tableString+="</tr>"
});
document.querySelector('#tb').innerHTML=tableString;
table td {
border:1px solid black;
}
<table id="tb">
</table>
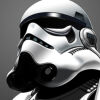
TA贡献1853条经验 获得超9个赞
您的代码基本上是正确的,但存在一些结构问题。您在函数内部定义函数会导致一些问题,您没有声明所有变量并且无法正确访问单元。看一下,如果您需要更多帮助,请告诉我。
let units = [
{
'code': 'COMP2110',
'title': 'Web Technology',
'offering': 'S1'
},
{
'code': 'COMP2010',
'title': 'Algorithms and Data Structures',
'offering': 'S1'
},
{
'code': 'COMP2150',
'title': 'Game Design',
'offering': 'S1'
},
{
'code': 'COMP2320',
'title': 'Offensive Security',
'offering': 'S1'
},
{
'code': 'COMP2200',
'title': 'Data Science',
'offering': 'S2'
},
{
'code': 'COMP2250',
'title': 'Data Communications',
'offering': 'S2'
},
{
'code': 'COMP2300',
'title': 'Applied Cryptography',
'offering': 'S2'
},
{
'code': 'COMP2000',
'title': 'Object-Oriented Programming Practices',
'offering': 'S2'
},
{
'code': 'COMP2050',
'title': 'Software Engineering',
'offering': 'S2'
},
{
'code': 'COMP2100',
'title': 'Systems Programming',
'offering': 'S2'
}
]
key=['code','title','offering'];
console.log(units[1].title);
var totalRows = 3;
var cellsInRow = 3;
var n=0,m=0;
function drawTable() {
console.log('draw');
// get the reference for the body
var first = document.getElementById('first');
// creates a <table> element
var tbl = document.createElement("table");
// creating rows
for (var r = 0; r < totalRows; r++) {
var row = document.createElement("tr");
// create cells in row
m=0;
for (var c = 0; c < cellsInRow; c++) {
var cell = document.createElement("td");
var cellText = document.createTextNode(units[n][key[m]]);
cell.appendChild(cellText);
row.appendChild(cell);
m=m+1;
}
n=n+1;
console.log(row);
tbl.appendChild(row); // add the row to the end of the table body
}
first.appendChild(tbl); // appends <table> into <first>
}
// your code here
window.onload=drawTable();
<div id='first'></div>
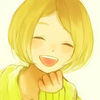
TA贡献1820条经验 获得超9个赞
您可以通过利用DOM API和Array.prototype.reduce
方法轻松实现这一点 - 例如:
const units = [{
'code': 'COMP2110',
'title': 'Web Technology',
'offering': 'S1'
},
{
'code': 'COMP2010',
'title': 'Algorithms and Data Structures',
'offering': 'S1'
},
{
'code': 'COMP2150',
'title': 'Game Design',
'offering': 'S1'
},
{
'code': 'COMP2320',
'title': 'Offensive Security',
'offering': 'S1'
},
{
'code': 'COMP2200',
'title': 'Data Science',
'offering': 'S2'
},
{
'code': 'COMP2250',
'title': 'Data Communications',
'offering': 'S2'
},
{
'code': 'COMP2300',
'title': 'Applied Cryptography',
'offering': 'S2'
},
{
'code': 'COMP2000',
'title': 'Object-Oriented Programming Practices',
'offering': 'S2'
},
{
'code': 'COMP2050',
'title': 'Software Engineering',
'offering': 'S2'
},
{
'code': 'COMP2100',
'title': 'Systems Programming',
'offering': 'S2'
}
];
const createEmptyTable = () => {
const tableEl = document.createElement('table');
tableEl.appendChild(document.createElement('thead'));
tableEl.appendChild(document.createElement('tbody'));
return tableEl;
};
const createTableHeadersRow = data => {
const fields = Object.keys(data);
return fields.reduce((trEl, fieldName) => {
const tdEl = document.createElement('th');
tdEl.appendChild(document.createTextNode(fieldName));
trEl.appendChild(tdEl);
return trEl;
}, document.createElement('tr'));
};
const createTableBodyRow = data => {
const values = Object.values(data);
return values.reduce((trEl, value) => {
const tdEl = document.createElement('td');
tdEl.appendChild(document.createTextNode(value));
trEl.appendChild(tdEl);
return trEl;
}, document.createElement('tr'));
};
const createUnitTable = unitsArray => {
return unitsArray.reduce((tableEl, unit, idx) => {
const tableNeedsHeaderRow = idx === 0;
if (tableNeedsHeaderRow) {
tableEl.querySelector('thead').appendChild(createTableHeadersRow(unit));
}
tableEl.querySelector('tbody').appendChild(createTableBodyRow(unit));
return tableEl;
}, createEmptyTable());
};
document.querySelector('div').appendChild(createUnitTable(units));
td {
padding: .5rem;
border: 1px solid black;
}
th {
text-transform: capitalize
}
<div></div>
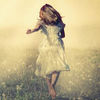
TA贡献1789条经验 获得超10个赞
对象数组示例
let cars = [
{
"color": "purple",
"type": "minivan",
"registration": new Date('2017-01-03'),
"capacity": 7
},
{
"color": "red",
"type": "station wagon",
"registration": new Date('2018-03-03'),
"capacity": 5
}]
功能 :
function ArrayToHtmlTable(htmlelement,ArrayObject) {
TableHeader = Object.keys(ArrayObject[0])
.map((x) => "<th>" + x + "</th>")
.join("");
TableBody = ArrayObject.map(
(x) =>
"<tr>" +
Object.values(x)
.map((x) => "<td>" + x + "</td>")
.join() +
"<tr>"
).join("");
document.getElementById(
htmlelement
).innerHTML += `<table> ${TableHeader} ${TableBody}</table>`;
}
函数调用:
ArrayToHtmlTable("testTable",cars)
- 4 回答
- 0 关注
- 143 浏览
添加回答
举报