4 回答
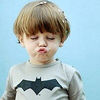
TA贡献1942条经验 获得超3个赞
假设数组中不能有布尔值,您可以执行以下操作:
<?php
/**
* @param string[] $standard
* @param string[] $to_compare
* @return bool
*/
function compare(array $standard, array $to_compare) : bool {
$standard_item = reset($standard);
foreach ($to_compare as $item) {
// Skip standard items assuming they are all optional
while ($item !== $standard_item) {
$standard_item = next($standard);
if ($standard_item === false) {
return false;
}
}
if ($standard_item === false || $item !== $standard_item) {
return false;
}
}
return true;
}
如果您想支持false标准数组中的值,可以修改上面的代码,以便通过索引引用项目,例如$standard[$i]。但这种方法也有其缺点——键必须是数字且连续的。对于更通用的解决方案,我可能会使用迭代器,例如ArrayIterator.
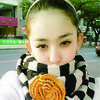
TA贡献1890条经验 获得超9个赞
我发现一个可能的解决方案很容易遵循:
class Transition
{
private string $fromValue;
private array $toValues;
public function __construct(string $fromValue, array $toValues)
{
$this->fromValue = $fromValue;
$this->toValues = $toValues;
}
public function getFromValue(): string
{
return $this->fromValue;
}
public function getToValues(): array
{
return $this->toValues;
}
}
function prepareTransitionsMap(array $orderDefinitions): array
{
$transitions = [];
$definitionsCount = count($orderDefinitions);
foreach ($orderDefinitions as $i => $fromValue) {
$toValues = [];
for ($j = $i; $j < $definitionsCount; ++$j) {
$toValues[] = $orderDefinitions[$j];
}
$transitions[$fromValue] = new Transition($fromValue, $toValues);
}
return $transitions;
}
function isArrayOrderValid(array $orderDefinitions, array $valuesToCheck): bool
{
$valuesCount = count($valuesToCheck);
if ($valuesCount === 0) {
return true;
}
$definitionsCount = count($orderDefinitions);
if ($definitionsCount === 0) {
return false;
}
$transitionsMap = prepareTransitionsMap($orderDefinitions);
foreach ($valuesToCheck as $i => $iValue) {
$valueToCheck = $iValue;
// value is no defined at all
if (!array_key_exists($valueToCheck, $transitionsMap)) {
return false;
}
// value is the last in the array
if (!array_key_exists($i + 1, $valuesToCheck)) {
return true;
}
$nextValue = $valuesToCheck[$i + 1];
$transition = $transitionsMap[$valueToCheck];
if (!in_array($nextValue, $transition->getToValues(), true)) {
return false;
}
}
return true;
}
isArrayOrderValid($standard, $valid_order); // true
isArrayOrderValid($standard, $invalid_order); // false
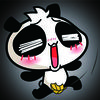
TA贡献1806条经验 获得超5个赞
你想使用usort()
https://www.php.net/manual/fr/function.usort.php
它看起来像
function custom_sort(&$my_array) {
return usort($my_array, function ($a, $b) {
global $etalon;
$a_key = array_search($a, $etalon);
$b_key = array_search($b, $etalon);
if (($a_key === FALSE) || ($b_key === FALSE) || ($a_key == $b_key)) {
return 0;
}
($a_key < $b_key) ? -1 : 1;
});
}
custom_sort($valid_order);
custom_sort($invalid_order)
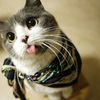
TA贡献1811条经验 获得超6个赞
另一个可以提供帮助的解决方案:
// Etalon
$etalon = array(
'taxonomy',
'post_meta',
'author',
'date',
'post_meta_num'
);
// Valid order
$valid_order = array(
'taxonomy',
'taxonomy',
'post_meta',
'date'
);
// Invalid order, 'author' is before 'post_meta'
$invalid_order = array(
'taxonomy',
'author',
'author',
'post_meta'
);
function checkOrder($array , $etalon)
{
$array = array_values(array_unique($array));
$array = array_intersect($array, $etalon );
foreach($array as $key => $value){
if(!in_array($array[$key],$etalon) || array_search($array[$key], $etalon)<$key){
return false;
}
}
return true;
}
var_dump(checkOrder($valid_order,$etalon)); // true
var_dump(checkOrder($invalid_order,$etalon)); // false
- 4 回答
- 0 关注
- 149 浏览
添加回答
举报