4 回答
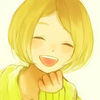
TA贡献1796条经验 获得超10个赞
const sorting = () => {
const images = document.getElementsByTagName('img');
const deck = [].slice.call(images);
const container = document.getElementById('cardShop');
const deckSorted = deck.sort((a, b) => {
const aToSort = a.getAttribute('value').split(',')[0];
const bToSort = b.getAttribute('value').split(',')[0];
return aToSort - bToSort;
});
for(let i = 0; i < deck.length; i++) {
deck[i].parentNode.removeChild(deck[i]);
container.append(deckSorted[i]);
}
}
<div id="cardShop" style="overflow:scroll">
<div id ="toSort" onclick="sorting()">Sort</div>
<img id="card" height="400" width="250" src="./cardImages/blueEyes.jpg" value = 3000,2500>
<img id="card2" height="400" width="250" src="./cardImages/blue.jpg" value = 1400,1200>
<img id="card3" height="400" width="250" src="./cardImages/orange.jpg" value = 1200,700>
<img id="card4" height="400" width="250" src="./cardImages/blackDrag.jpg" value = 3200,2500>
<img id="card5" height="400" width="250" src="./cardImages/spider.jpg" value = 2500,2100>
<img id="card6" height="400" width="250" src="./cardImages/dark.jpg" value = 2500,2100>
<img id="card7" height="400" width="250" src="./cardImages/grill.jpg" value = 2000,1700>
<img id="card8" height="400" width="250" src="./cardImages/gaia.jpg" value = 2300,2100>
<img id="card9" height="400" width="250" src="./cardImages/redEyes.jpg" value = 2400,2000>
<img id="card10" height="400" width="250" src="./cardImages/flamee.jpg" value = 1800,1600>
<img id="card11" height="400" width="250" src="./cardImages/curse.jpg" value = 2800,2200>
<img id="card12" height="400" width="250" src="./cardImages/tripple.jpg" value = 4500,3800>
</div>
您可以使用排序来完成此操作,然后删除旧元素并附加新元素,如下所示。
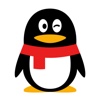
TA贡献1725条经验 获得超7个赞
const setup = () => {
const toSort = document.querySelector('#toSort');
toSort.addEventListener('click', sorting);
};
const sorting = () => {
let cardShop = document.querySelector('#cardShop');
let dek = [...cardShop.querySelectorAll('img')]
.sort((a,b) => {
let valuesA = a.getAttribute('value').split(',');
let valuesB = b.getAttribute('value').split(',');
let comparer = valuesA[0].localeCompare(valuesB[0]);
if(comparer === 0)
comparer = valuesA[1].localeCompare(valuesB[1]);
return comparer;
})
.map(node=>cardShop.appendChild(node));
};
//load
window.addEventListener('load', setup);
#toSort{
background-color: #3b5998;
width:50px;
height:50px;
float:right;
}
<!DOCTYPE html>
<html>
<body>
<div id = "cardShop" style="overflow:scroll">
<div id ="toSort"></div>
<img id="card" height="400" width="250" src="./cardImages/blueEyes.jpg" value="3000,2500">
<img id="card2" height="400" width="250" src="./cardImages/blue.jpg" value="1400,1200">
<img id="card3" height="400" width="250" src="./cardImages/orange.jpg" value="1200,700">
<img id="card4" height="400" width="250" src="./cardImages/blackDrag.jpg" value="3200,2500">
<img id="card5" height="400" width="250" src="./cardImages/spider.jpg" value="2500,2100">
<img id="card6" height="400" width="250" src="./cardImages/dark.jpg" value="2500,2100">
<img id="card7" height="400" width="250" src="./cardImages/grill.jpg" value="2000,1700">
<img id="card8" height="400" width="250" src="./cardImages/gaia.jpg" value="2300,2100">
<img id="card9" height="400" width="250" src="./cardImages/redEyes.jpg" value="2400,2000">
<img id="card10" height="400" width="250" src="./cardImages/flamee.jpg" value="1800,1600">
<img id="card11" height="400" width="250" src="./cardImages/curse.jpg" value="2800,2200">
<img id="card12" height="400" width="250" src="./cardImages/tripple.jpg" value="4500,3800">
</div>
</body>
</html>
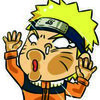
TA贡献1936条经验 获得超6个赞
你走在正确的道路上,我为你完成了代码。该代码将所有图像推送到一个包含 json 元素的数组,该数组包含元素和值(左侧)。然后,它按正确的顺序对它们进行排序,删除所有图像并按正确的顺序再次插入它们。
工作示例: https: //playcode.io/537993
function sorting() {
let deck = [].slice.call(document.getElementById("cardShop").children)
let images = []
let button = document.getElementById('toSort');
for (let i = 1; i < deck.length; i++) {
let elementValue = deck[i].getAttribute('value').split(",").map(Number);
// add all values to a array with json info about the element
images.push({
'element': deck[i],
'value': elementValue[0]
});
}
// sort them on the value
images.sort((a, b) => {
return a.value - b.value;
})
// remove all elements in the div
document.getElementById('cardShop').innerHTML = '';
// add the button again
document.getElementById('cardShop').appendChild(button);
// Add the images again
images.forEach(image => {
document.getElementById('cardShop').appendChild(image.element)
})
}
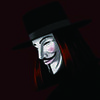
TA贡献1836条经验 获得超13个赞
您的 HTML 标记已损坏。确保将其重构为<img ... value="1200,2500" />而不是<img ... value=1 200,2500 />,因为 Javascript 无法处理它。
function sorting () {
const imgWrapper = document.getElementById('cardShop')
const deck = [].slice.call(imgWrapper.getElementsByTagName('img'))
deck.sort((currentImage, nextImage) => {
const currentImageValue = parseInt(currentImage.getAttribute('value').split(',')[0])
const nextImageValue = parseInt(nextImage.getAttribute('value').split(',')[0])
return currentImageValue - nextImageValue
})
// at this point the array has been sorted, but not the actual HTML!
console.log(deck)
// optional - if you want to redraw them with the new order in the HTML
const oldImgHTMLCollection = [].slice.call(imgWrapper.getElementsByTagName('img'))
oldImgHTMLCollection.forEach(oldImg => imgWrapper.removeChild(oldImg))
deck.forEach(newImg => imgWrapper.appendChild(newImg))
}
sorting()
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Document</title>
<script src="script.js"></script>
<style>
</style>
</head>
<body>
<div id="cardShop" style="overflow:scroll">
<div id="toClose"></div>
<div id="toSort"></div>
<img id="card" height="400" width="250" src="./cardImages/blueEyes.jpg" value="3000,2500">
<img id="card2" height="400" width="250" src="./cardImages/blue.jpg" value="1400,1200">
<img id="card3" height="400" width="250" src="./cardImages/orange.jpg" value="1200,700">
<img id="card4" height="400" width="250" src="./cardImages/blackDrag.jpg" value="3200,2500">
<img id="card5" height="400" width="250" src="./cardImages/spider.jpg" value="2500,2100">
<img id="card6" height="400" width="250" src="./cardImages/dark.jpg" value="2500,2100">
<img id="card7" height="400" width="250" src="./cardImages/grill.jpg" value="2000,1700">
<img id="card8" height="400" width="250" src="./cardImages/gaia.jpg" value="2300,2100">
<img id="card9" height="400" width="250" src="./cardImages/redEyes.jpg" value="2400,2000">
<img id="card10" height="400" width="250" src="./cardImages/flamee.jpg" value="1800,1600">
<img id="card11" height="400" width="250" src="./cardImages/curse.jpg" value="2800,2200">
<img id="card12" height="400" width="250" src="./cardImages/tripple.jpg" value="4500,3800">
</div>
</body>
</html>
- 4 回答
- 0 关注
- 152 浏览
添加回答
举报