3 回答
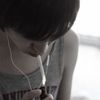
TA贡献1797条经验 获得超6个赞
所以我想你想要一个按钮来跳过图像和一个按钮来显示上一张图像。
首先我们声明变量
const img = document.getElementById("image"); // the img element
const imageSources = ["image1.png", "image2.png","image3.png"];
let index = 0;
let travel; // here the most important thing
您必须创建一个启动函数,以便您可以在页面加载或重置计时器时调用它
const startTravel = () => {
travel = setInterval(() => {
++index;
if (index < 0 || index === imageSources.length) index = 0;
img.src = imageSources[index];
}, 2000);
};
下一个图像函数就像
const changeImage2 = () => {
clearInterval(travel);
img.src = imageSources[++index];
startTravel();
}
之前的图像函数就像
const changeImage1 = () => {
clearInterval(travel);
img.src = imageSources[--index];
startTravel();
}
它还没有完成,当你点击跳过 5 次时,它会将索引设置为 6,而 6 不在 imageSources 中,所以我不想为你做这件事,因为它很容易,我认为你必须做到这一点
完整代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<button id="button1" onclick="changeImage1()">
left
</button>
<img src="image1.jpg" alt="img" id="img">
<button id="button2" onclick="changeImage2()">
right
</button>
<script>
const img = document.getElementById("img");
const imageSources = ["image1.jpg", "image2.jpg", "image3.jpg"];
let index = 0;
let travel;
const startTravel = () => {
travel = setInterval(() => {
++index;
console.log(index);
if (index < 0 || index === imageSources.length) index = 0;
img.src = imageSources[index];
}, 6000);
};
const changeImage1 = () => {
if (index === 0) index = imageSources.length;
clearInterval(travel);
img.src = imageSources[--index];
startTravel();
}
const changeImage2 = () => {
if (index === imageSources.length - 1) index = -1;
clearInterval(travel);
img.src = imageSources[++index];
startTravel();
}
startTravel();
</script>
</body>
</html>
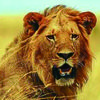
TA贡献1803条经验 获得超3个赞
您正在寻找类似于滑块的功能。您显示的第一个片段setInterval是一个很好的工作场所。迭代列表使您的代码变得动态,并允许添加或删除项目,而无需更改太多代码。
我扩展了您提供的代码,并在下面制作了一个可行的代码片段。一定要检查一下。
而不是setInterval我用过的setTimeout. 这样做的原因是因为按钮和循环使用相同的功能来显示当前图像。所以循环将能够再次调用自身。当循环在 2 秒过去之前被调用时,它将被取消并重新开始。当您单击其中一个按钮时可能会发生这种情况。
我已经删除了内联onclick侦听器,转而addEventListener在 JavaScript 中使用。我建议你采用这种方法。它将允许您重复更少的代码,将 JavaScript 保留在一个地方,并使您的代码更加灵活。
不过,我确实value向按钮添加了一个属性。按钮支持此属性。它可以携带少量信息,例如告诉您此按钮是上一个按钮还是下一个按钮。该属性可以通过对象的属性(相当于 JS 中的 a )value轻松读取。valueHTMLButtonElement<button>
如果您有任何问题,请务必提出,因为我认为有些事情对您来说可能是新的。
// The element to display the image with.
const imageElement = document.querySelector('.js-image-display');
// The buttons to go previous and next.
const imageButtons = document.querySelectorAll('.js-image-control');
// Available sources of images.
const imageSources = [
"https://www.placecage.com/c/800/300",
"https://www.placecage.com/800/300",
"https://www.fillmurray.com/800/300",
"https://www.placecage.com/g/800/300",
"https://www.fillmurray.com/g/800/300"
];
// Current displayed image.
let currentImageIndex = 0;
// Variable to store the loop in.
let currentLoop;
// Show first image and start looping.
showCurrentImage();
/**
* Cancel previous loop, wait for 2 seconds and call nextImage().
* nextImage() will then call showCurrentImage which will call
* loop() again. This will keep the cycle going.
*/
function loop() {
clearTimeout(currentLoop);
currentLoop = setTimeout(nextImage, 2000);
}
/**
* Update the src of the imageElement with the
* current image index. Then reset the loop.
*/
function showCurrentImage() {
imageElement.src = imageSources[currentImageIndex];
loop();
}
/**
* Remove 1 from the current image index
* or go back to the end. Then show the image.
*/
function prevImage() {
if (currentImageIndex === 0) {
currentImageIndex = imageSources.length - 1;
} else {
currentImageIndex--;
}
showCurrentImage();
}
/**
* Add 1 to current image index or go
* back to the start. Then show the image.
*/
function nextImage() {
if (currentImageIndex === imageSources.length - 1) {
currentImageIndex = 0;
} else {
currentImageIndex++;
}
showCurrentImage();
}
// Link the prev and next words to their corresponding functions.
// This way you don't have to write a lot of if / else statements
// to get the function based on the value of the button.
const actionMap = {
'prev': prevImage,
'next': nextImage
}
/**
* Decide by reading the value attribute if nextImage or
* prevImage should be called. event.currentTarget is one
* of the two buttons that you've clicked. It gets the value
* and looks up the function to call from the actionMap.
*
* @param {Event} event Click event triggerd by the buttons.
*/
function onButtonClick(event) {
const value = event.currentTarget.value;
const action = actionMap[value];
action();
}
// Loop over the buttons and add an click event listener
// for each button in the list. In some older browser imageButtons
// might not be able to use forEach, so Array.from() turns it
// into an array so we know for sure that forEach is possible.
Array.from(imageButtons).forEach(function(button) {
button.addEventListener('click', onButtonClick);
});
img {
max-width: 100%;
height: auto;
}
<!-- Prev button -->
<button class="js-image-control" value="prev">Previous</button>
<!-- Image -->
<img class="js-image-display" src="image1.png" width="800" height="300"/>
<!-- Next button -->
<button class="js-image-control" value="next">Next</button>
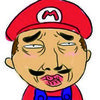
TA贡献1851条经验 获得超5个赞
事情是,
if (index === imageSources.length) {
index = 0;
}
这行代码或三行代码等待 imageSources 长度变为 3,但 imageSources 从 1 开始计数,因此如果 imageSources 变量包含 3 个元素,则长度将为 3。然而索引是由 0 完成的,所以你必须将代码更改为
if (index === (imageSources.length - 1)) {
index = 0;
}
添加回答
举报