1 回答
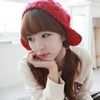
TA贡献1876条经验 获得超7个赞
按钮的默认类型是submit这样,只需添加type='button'“添加位置按钮”即可解决您的问题
<!DOCTYPE html>
<html>
<head>
<!-- BootStrap 4 CSS -->
<link
rel="stylesheet"
href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm"
crossorigin="anonymous"
/>
<!-- Jquery -->
<script
src="https://code.jquery.com/jquery-3.2.1.slim.min.js"
integrity="sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN"
crossorigin="anonymous"
></script>
<!-- BootStrap 4 JS -->
<script
src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"
integrity="sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl"
crossorigin="anonymous"
></script>
<link rel="stylesheet" type="text/css" href="./index.css" />
<script type="text/javascript">
var numPlaces = 4;
function addPlace() {
numPlaces++;
console.log(numPlaces);
var place = document.createElement("DIV");
place.id = "p" + numPlaces;
var group1 = document.createElement("DIV");
group1.className = "form-group";
var label1 = document.createElement("LABEL");
label1.className = "custom-control-label";
label1.style.fontWeight = "bold";
label1.innerHTML = "Place " + numPlaces;
var address = document.createElement("INPUT");
address.type = "text";
address.className = "form-control";
address.id = "place" + numPlaces;
address.placeholder = "Enter Address of Place you Visited";
group1.appendChild(label1);
group1.appendChild(address);
var group2 = document.createElement("DIV");
group2.className = "form-group";
var label2 = document.createElement("LABEL");
label2.className = "custom-control-label";
label2.innerHTML = "Enter the day you visited this place.";
var date = document.createElement("INPUT");
date.type = "date";
date.className = "form-control";
date.id = "date" + numPlaces;
group2.appendChild(label2);
group2.appendChild(date);
var group3 = document.createElement("DIV");
group3.className = "form-group";
var label3 = document.createElement("LABEL");
label3.className = "custom-control-label";
label3.innerHTML =
"Did you visit in the morning (A.M. times) or the afternoon (P.M. times)?";
var amDiv = document.createElement("DIV");
amDiv.className = "custom-control custom-radio custom-control-inline";
var amInput = document.createElement("INPUT");
amInput.type = "radio";
amInput.className = "custom-control-input";
amInput.id = "am" + numPlaces;
amInput.name = "time" + numPlaces;
var label4 = document.createElement("LABEL");
label4.className = "custom-control-label";
label4.for = amInput.id;
label4.innerHTML = "Morning";
amDiv.appendChild(amInput);
amDiv.appendChild(label4);
var pmDiv = document.createElement("DIV");
pmDiv.className = "custom-control custom-radio custom-control-inline";
var pmInput = document.createElement("INPUT");
pmInput.type = "radio";
pmInput.className = "custom-control-input";
pmInput.id = "pm" + numPlaces;
pmInput.name = "time" + numPlaces;
var label5 = document.createElement("LABEL");
label5.className = "custom-control-label";
label5.for = pmInput.id;
label5.innerHTML = "Afternoon";
pmDiv.appendChild(pmInput);
pmDiv.appendChild(label5);
group3.appendChild(amDiv);
group3.appendChild(pmDiv);
place.appendChild(group1);
place.appendChild(group2);
place.appendChild(group3);
document.getElementById("addedPlaces").appendChild(place);
}
</script>
<title>Testing App</title>
</head>
<body>
<div class="container">
<nav class="navbar navbar-expand-lg navbar-light bg-light fixed-top">
<a class="navbar-brand" href="#">Am I At Risk?</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNavDropdown" aria-controls="navbarNavDropdown" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNavDropdown">
<ul class="navbar-nav">
<li class="nav-item active">
<a class="nav-link" href="userForm.php">Enter Details<span class="sr-only">(current)</span></a>
</li>
<li class="nav-item">
<a class="nav-link" href="governmentForm.php">Form for Government</a>
</li>
</ul>
</div>
</nav>
<br /><br /><br />
<form>
<div class="form-group">
<label>Please enter the various places you visited in the past 14 days.</label>
</div>
<div id="p1">
<div class="form-group">
<label class="custom-control-label"><b>Place 1</b></label>
<input type="text" class="form-control" id="place1" placeholder="Enter Address of Place you Visited"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Enter the day you visited this place.</label>
<input type="date" class="form-control" id="date1"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Did you visit in the morning (A.M. times) or the afternoon (P.M. times)?</label>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="am1" name="time1" checked>
<label class="custom-control-label" for="am1">Morning</label>
</div>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="pm1" name="time1" checked>
<label class="custom-control-label" for="pm1">Afternoon</label>
</div>
</div>
</div>
<br />
<div id="p2">
<div class="form-group">
<label class="custom-control-label"><b>Place 2</b></label>
<input type="text" class="form-control" id="place2" placeholder="Enter Address of Place you Visited"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Enter the day you visited this place.</label>
<input type="date" class="form-control" id="date2"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Did you visit in the morning (A.M. times) or the afternoon (P.M. times)?</label>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="am2" name="time2" checked>
<label class="custom-control-label" for="am2">Morning</label>
</div>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="pm2" name="time2" checked>
<label class="custom-control-label" for="pm2">Afternoon</label>
</div>
</div>
</div>
<br />
<div id="p3">
<div class="form-group">
<label class="custom-control-label"><b>Place 3</b></label>
<input type="text" class="form-control" id="place3" placeholder="Enter Address of Place you Visited"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Enter the day you visited this place.</label>
<input type="date" class="form-control" id="date3"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Did you visit in the morning (A.M. times) or the afternoon (P.M. times)?</label>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="am3" name="time3" checked>
<label class="custom-control-label" for="am3">Morning</label>
</div>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="pm3" name="time3" checked>
<label class="custom-control-label" for="pm3">Afternoon</label>
</div>
</div>
</div>
<br />
<div id="p4">
<div class="form-group">
<label class="custom-control-label"><b>Place 4</b></label>
<input type="text" class="form-control" id="place4" placeholder="Enter Address of Place you Visited"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Enter the day you visited this place.</label>
<input type="date" class="form-control" id="date4"></input>
</div>
<div class="form-group">
<label class="custom-control-label">Did you visit in the morning (A.M. times) or the afternoon (P.M. times)?</label>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="am4" name="time4" checked>
<label class="custom-control-label" for="am4">Morning</label>
</div>
<div class="custom-control custom-radio custom-control-inline">
<input type="radio" class="custom-control-input" id="pm4" name="time4" checked>
<label class="custom-control-label" for="pm4">Afternoon</label>
</div>
</div>
</div>
<br />
<div id="addedPlaces"></div>
<button type="button" onclick="addPlace();">Add Place</button>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
<!-- end of feature div -->
</body>
</html>
- 1 回答
- 0 关注
- 59 浏览
添加回答
举报