1 回答
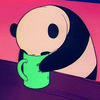
TA贡献1934条经验 获得超2个赞
使用 aSemaphore很可能不是正确的方法。您想要的是进入嵌套事件循环,而不是使用阻塞机制。从阅读 API 来看,你似乎把事情过于复杂化了。同样,您所需要的只是在一个 UI 线程上进入嵌套事件循环,然后在另一个 UI 线程完成其工作后退出该循环。我相信以下内容可以满足您的要求:
import java.awt.EventQueue;
import java.awt.SecondaryLoop;
import java.awt.Toolkit;
import java.util.Objects;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.Supplier;
import javafx.application.Platform;
import javax.swing.SwingUtilities;
public class Foo {
public static <T> T getOnFxAndWaitOnEdt(Supplier<? extends T> supplier) {
Objects.requireNonNull(supplier, "supplier");
if (!EventQueue.isDispatchThread()) {
throw new IllegalStateException("current thread != EDT");
}
final SecondaryLoop loop = Toolkit.getDefaultToolkit()
.getSystemEventQueue()
.createSecondaryLoop();
final AtomicReference<T> valueRef = new AtomicReference<>();
Platform.runLater(() -> {
valueRef.set(supplier.get());
SwingUtilities.invokeLater(loop::exit);
});
loop.enter();
return valueRef.get();
}
public static <T> T getOnEdtAndWaitOnFx(Supplier<? extends T> supplier) {
Objects.requireNonNull(supplier, "supplier");
if (!Platform.isFxApplicationThread()) {
throw new IllegalStateException(
"current thread != JavaFX Application Thread");
}
final Object key = new Object();
final AtomicReference<T> valueRef = new AtomicReference<>();
SwingUtilities.invokeLater(() -> {
valueRef.set(supplier.get());
Platform.runLater(() -> Platform.exitNestedEventLoop(key, null));
});
Platform.enterNestedEventLoop(key);
return valueRef.get();
}
}
JavaFX 9 中添加了和方法,尽管 JavaFX 8 中也有等效的内部方法。使用的原因是因为在 lambda 表达式内部使用时,局部变量必须是 Final 或有效的 Final Platform#enterNestedEventLoop。然而,由于通知单独线程的方式,我不认为 和方法提供的易变性语义是严格需要的,但我使用了这些方法以防万一。Platform#exitNestedEventLoopAtomicReference#get()#set(T)AtomicReference
以下是使用上述内容从事件调度线程显示模式 JavaFX 对话框的示例:
Optional<T> optional = Foo.getOnFxAndWaitOnEdt(() -> {
Dialog<T> dialog = new Dialog<>();
// configure dialog...
return dialog.showAndWait();
});
上述实用程序方法用于从事件调度线程到JavaFX 应用程序线程进行通信,反之亦然。这就是为什么需要输入嵌套事件循环,否则 UI 线程之一将必须阻塞,从而冻结关联的 UI。如果您位于非 UI 线程上并且需要在等待结果时在 UI 线程上运行操作,则解决方案要简单得多:
// Run on EDT
T result = CompletableFuture.supplyAysnc(/*Supplier*/, SwingUtilities::invokeLater).join();
// Run on FX thread
T result = CompletableFuture.supplyAsync(/*Supplier*/, Platform::runLater).join();
调用join()将阻塞调用线程,因此请确保不要从任一 UI 线程调用该方法。
添加回答
举报