2 回答
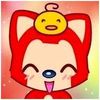
TA贡献1864条经验 获得超6个赞
您的数据有一个小问题,Ghost并且Car具有相同的 ID。修复此问题后,您可以使用以下代码创建结果数组:
const products = [{
id: "A32S",
title: "Car"
},
{
id: "D12E",
title: "Rabbit"
},
{
id: "A33S",
title: "Ghost"
},
{
id: "34SC",
title: "Apple"
},
];
const newProducts = [{
id: "A32S",
title: "Ferrari"
},
{
id: "D12E",
title: "Rabbit"
},
{
id: "A33S",
title: "Ghost"
}
]
const changes = [];
function hasSameValue(product, otherProduct, key) {
return product[key] === otherProduct[key];
}
function exists(product, productArray) {
for (const existingProduct of productArray) {
if (hasSameValue(product, existingProduct, "id")) return true;
}
return false;
}
function getExistingProduct(id, productArray) {
for (const product of productArray) {
if (product.id === id) return product;
}
}
for (const product of newProducts) {
if (exists(product, products)) {
const existingProduct = getExistingProduct(product.id, products);
if (!hasSameValue(product, existingProduct, "title")) {
changes.push(product);
}
}
}
for (const product of products) {
if (!exists(product, newProducts)) {
changes.push({
id: product.id,
removed: true
});
}
}
console.log(changes);
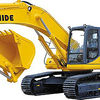
TA贡献1829条经验 获得超7个赞
如果您想获取所有更改(添加、删除、更新和未更改)以及检查对象的多个键,您也可以尝试类似的操作。
时间复杂度为 O(N),空间复杂度为 O(N)。假设“id”是唯一的。
function getProductChanges(oldProductsList, newProductsList) {
let oldProductsMap = {};
let newProductsMap = {};
let addedProductsList = [];
let updatedProductsList = [];
let removedProductsList = [];
let unchangedProductsList = [];
// considering id is unique
// creating map for old products list
oldProductsList.map((oldProduct) => {
oldProductsMap[oldProduct.id] = oldProduct;
});
// creating map for new products list and
// filtering newly added, updated and unchanged products list
newProductsList.map((newProduct) => {
newProductsMap[newProduct.id] = newProduct;
let oldProduct = oldProductsMap[newProduct.id];
if (oldProduct) {
// if an existing product is updated
if (JSON.stringify(oldProduct) !== JSON.stringify(newProduct)) {
updatedProductsList.push(newProduct);
} else {
unchangedProductsList.push(newProduct);
}
}
else {
// if a new product is added
addedProductsList.push(newProduct);
}
});
// populating removedProductsList
removedProductsList = oldProductsList.filter((oldProduct) => !newProductsMap[oldProduct.id]);
return {
added: addedProductsList,
updated: updatedProductsList,
removed: removedProductsList,
unchanged: unchangedProductsList,
};
}
输入:
let products = [
{ id: "A32S", title: "Car" },
{ id: "D12E", title: "Rabbit" },
{ id: "A321", title: "Ghost" },
{ id: "A320", title: "Lion" },
];
let newProducts = [
{ id: "A32S", title: "Ferrari" },
{ id: "D12E", title: "Rabbit" },
{ id: "A321", title: "Ghost" },
{ id: "A322", title: "Zebra" },
];
console.log(getProductChanges(products, newProducts));
输出:
{
added: [{ id: "A322", title: "Zebra" }],
removed: [{ id: "A320", title: "Lion" }],
unchanged: [
{ id: "D12E", title: "Rabbit" },
{ id: "A321", title: "Ghost" },
],
updated: [{ id: "A32S", title: "Ferrari" }],
}
添加回答
举报