3 回答
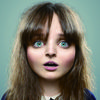
TA贡献1794条经验 获得超7个赞
您可以将所有整数添加到 a 中List<Integer>,对其进行排序,然后获取它的 X 最大个数:
public class foo {
public static void main(String[] args) {
int row = 3, col = 3, dep = 3;
int[][][] value = new int[row][col][dep];
value[1][2][1] = 10;
value[1][0][1] = 15;
List<Integer> listWithAll = new ArrayList<>();
/* insert the value from user or initialize the matrix*/
int max = 0;
for (row = 0; row < 3; row++)
for (col = 0; col < 3; col++)
for (dep = 0; dep < 3; dep++)
listWithAll.add(value[row][col][dep]);
listWithAll.sort(Collections.reverseOrder());
for (int i = 0; i < 10; i++) {
System.out.println(listWithAll.get(i));
}
}
}
打印:
15 10 0 0 0 0 0 0 0 0
或仅使用 Java 8 流:
List<Integer> max10 = listWithAll.stream()
.sorted(Collections.reverseOrder())
.limit(10)
.collect(Collectors.toList());
max10.forEach(System.out::println);
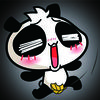
TA贡献1806条经验 获得超5个赞
这是一种使用 来做到这一点的方法streams。我使用了完整的 2 x 2 x 2 数组以使其更容易,但它适用于任何int[][][]数组。我没有使用嵌套循环,而是只使用flatMapped数组。
初始化数组。
int[][][] v = {{{1, 2}, {3, 4}}, {{5, 6}, {7, 8}}};
现在获取前 4 个值(或前 N 个值)并将它们放入列表中。
int top4 = 4;
List<Integer> top4Max =
Arrays.stream(v).flatMap(Arrays::stream).flatMapToInt(
Arrays::stream).boxed().sorted(
Comparator.reverseOrder()).limit(top4).collect(
Collectors.toList());
System.out.println(top4Max);
印刷
8 7 6 5
剥离Arrays.stream一层数组。将 flatMap它们进一步扁平化为数组single dimenion。将flatMapToInt其扁平化,将stream of ints其分类并加工成有限的集合。
如果需要,您还可以将它们放入数组而不是列表中。
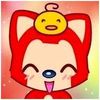
TA贡献1864条经验 获得超6个赞
或者,可以创建一个小数组并将其与普通的 findmax 函数一起使用。
public class Array_3D {
public static void main(String[] args) {
int row = 3, col = 3, dep = 3;
int[][][] value = new int[row][col][dep];
value[1][2][1] = 10;
value[1][0][1] = 15;
int[] topten = new int[10]; //array to hold max values
int count = 0;
int candidate = 0;
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
for (int k = 0; k < dep; k++) {
if (count < 10) { //start with first ten values
topten[count] = value[i][j][k];
count++;
}
else {
candidate = value[i][j][k];
for (int x = 0; x < 10; x++) { //loop for loading top values
if (candidate > topten[x]) {
topten[x] = candidate;
break; //exit on first hit
}
}
}
}
}
}
for (int i = 0; i < 10; i++) {
System.out.print(topten[i] + " ");
}
System.out.println();
}
}
我不知道对于大型 3D 数组哪种方法更快;这里我们必须在 3D 数组中的每个点运行一个小循环,而不是创建一个全新的列表并对其进行排序。显然,这在内存使用方面获胜,但不确定速度。[编辑注意,这将按原样返回前十个值的未排序数组]。
添加回答
举报