1 回答
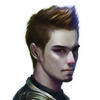
TA贡献1993条经验 获得超5个赞
我为此使用了类型安全枚举模式,我认为它适合您。通过 TypeSafeEnum,您可以使用 Newtonsoft 的 JsonConverter 属性控制映射到 JSON 的内容。由于您没有要发布的代码,我已经构建了一个示例。
应用程序的 TypeSafeEnums 使用的基类:
public abstract class TypeSafeEnumBase
{
protected readonly string Name;
protected readonly int Value;
protected TypeSafeEnumBase(int value, string name)
{
this.Name = name;
this.Value = value;
}
public override string ToString()
{
return Name;
}
}
作为 TypeSafeEnum 实现的示例类型,它通常是一个普通的 Enum,包括 Parse 和 TryParse 方法:
public sealed class BirdType : TypeSafeEnumBase
{
private const int BlueBirdId = 1;
private const int RedBirdId = 2;
private const int GreenBirdId = 3;
public static readonly BirdType BlueBird =
new BirdType(BlueBirdId, nameof(BlueBird), "Blue Bird");
public static readonly BirdType RedBird =
new BirdType(RedBirdId, nameof(RedBird), "Red Bird");
public static readonly BirdType GreenBird =
new BirdType(GreenBirdId, nameof(GreenBird), "Green Bird");
private BirdType(int value, string name, string displayName) :
base(value, name)
{
DisplayName = displayName;
}
public string DisplayName { get; }
public static BirdType Parse(int value)
{
switch (value)
{
case BlueBirdId:
return BlueBird;
case RedBirdId:
return RedBird;
case GreenBirdId:
return GreenBird;
default:
throw new ArgumentOutOfRangeException(nameof(value), $"Unable to parse for value, '{value}'. Not found.");
}
}
public static BirdType Parse(string value)
{
switch (value)
{
case "Blue Bird":
case nameof(BlueBird):
return BlueBird;
case "Red Bird":
case nameof(RedBird):
return RedBird;
case "Green Bird":
case nameof(GreenBird):
return GreenBird;
default:
throw new ArgumentOutOfRangeException(nameof(value), $"Unable to parse for value, '{value}'. Not found.");
}
}
public static bool TryParse(int value, out BirdType type)
{
try
{
type = Parse(value);
return true;
}
catch
{
type = null;
return false;
}
}
public static bool TryParse(string value, out BirdType type)
{
try
{
type = Parse(value);
return true;
}
catch
{
type = null;
return false;
}
}
}
用于处理类型安全转换的容器,因此您无需为实现的每个类型安全创建转换器,并在实现新的类型安全枚举时防止 TypeSafeEnumJsonConverter 发生更改:
public class TypeSafeEnumConverter
{
public static object ConvertToTypeSafeEnum(string typeName, string value)
{
switch (typeName)
{
case "BirdType":
return BirdType.Parse(value);
//case "SomeOtherType": // other type safe enums
// return // some other type safe parse call
default:
return null;
}
}
}
实现 Newtonsoft 的 JsonConverter,它又调用我们的 TypeSafeEnumConverter
public class TypeSafeEnumJsonConverter : JsonConverter
{
public override bool CanConvert(Type objectType)
{
var types = new[] { typeof(TypeSafeEnumBase) };
return types.Any(t => t == objectType);
}
public override object ReadJson(JsonReader reader, Type objectType, object existingValue, JsonSerializer serializer)
{
string name = objectType.Name;
string value = serializer.Deserialize(reader).ToString();
return TypeSafeEnumConversion.ConvertToTypeSafeEnum(name, value); // call to our type safe converter
}
public override void WriteJson(JsonWriter writer, object value, JsonSerializer serializer)
{
if (value == null && serializer.NullValueHandling == NullValueHandling.Ignore)
{
return;
}
writer.WriteValue(value?.ToString());
}
}
使用 BirdType 并设置要使用的转换器的示例对象:
public class BirdCoup
{
[JsonProperty("bird-a")]
[JsonConverter(typeof(TypeSafeEnumJsonConverter))] // sets the converter used for this type
public BirdType BirdA { get; set; }
[JsonProperty("bird-b")]
[JsonConverter(typeof(TypeSafeEnumJsonConverter))] // sets the converter for this type
public BirdType BirdB { get; set; }
}
使用示例:
// sample #1, converts value with spaces to BirdTyp
string sampleJson_1 = "{\"bird-a\":\"Red Bird\",\"bird-b\":\"Blue Bird\"}";
BirdCoup resultSample_1 =
JsonConvert.DeserializeObject<BirdCoup>(sampleJson_1, new JsonConverter[]{new TypeSafeEnumJsonConverter()});
// sample #2, converts value with no spaces in name to BirdType
string sampleJson_2 = "{\"bird-a\":\"RedBird\",\"bird-b\":\"BlueBird\"}";
BirdCoup resultSample_2 =
JsonConvert.DeserializeObject<BirdCoup>(sampleJson_2, new JsonConverter[] { new TypeSafeEnumJsonConverter() });
- 1 回答
- 0 关注
- 111 浏览
添加回答
举报