3 回答
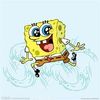
TA贡献1809条经验 获得超8个赞
您正在使用 Java 序列化,它将对象写入其自己的二进制格式,而不是文本。如果您需要文本格式,我建议您使用 JSON 以及jackson-databind等库。
为了方便起见,这里是一个工作示例(既写入文本文件又从文本文件读回对象):
主类
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import com.fasterxml.jackson.core.JsonGenerationException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) throws JsonGenerationException, JsonMappingException, FileNotFoundException, IOException {
ObjectMapper om = new ObjectMapper();
Test test = new Test("Short name of test","Full name of test");
om.writeValue(new FileOutputStream("test.json"), test);
Test readValue = om.readValue(new FileInputStream("test.json"), Test.class);
System.out.println(readValue.getShortName());
System.out.println(readValue.getFullName());
}
}
测试类
import java.io.Serializable;
public class Test implements Serializable{
private static final long serialVersionUID = 1L;
private String shortName;
private String fullName;
public Test() {
}
public Test(String shortName, String fullName) {
this.shortName=shortName;
this.fullName=fullName;
}
public String getShortName() {
return shortName;
}
public void setShortName(String shortName) {
this.shortName = shortName;
}
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
@Override
public String toString() {
return "Name:" + shortName + "\nFullName: " + fullName;
}
}
请注意,我已向 Test.class 添加了一个默认构造函数,以便 Jackson 可以在从 json 反序列化时创建它。
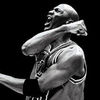
TA贡献1847条经验 获得超11个赞
writeObject 函数将 Test 的对象图写入文件流。在JAVA中用于持久化。如果您想将字段数据存储到文件中,我建议您在类中使用 FileWriter 的专用方法。
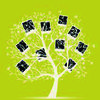
TA贡献1811条经验 获得超5个赞
ObjectOutputStream不会以可读格式写入。您可能想使用FileWriter:
try (BufferedWriter writer = new BufferedWriter(new FileWriter("test.txt"))) {
Test t = new Test("Short name of test", "Full name of test");
writer.write(t.toString());
} catch (IOException e) {
e.printStackTrace();
}
添加回答
举报