3 回答
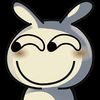
TA贡献2037条经验 获得超6个赞
您可以通过做一些数学运算、几个基本循环以及使用printvs来避免计算逻辑println。首先,我将定义此处使用的变量:
//constants
int DIGITS_PER_LINE = 10; //your number of digits per line
int DIGITS_OFFSET = 2; //always 2, because 0 = '3' and 1 = '.'
String pi = /* your PI string */;
//input
int decimals = 42; //for example, from the user
然后,使用循环打印结果:
//We can calculate the number of lines we need from the start
int lines = decimals / DIGITS_PER_LINE; //4, to start
lines += (decimals % DIGITS_PER_LINE > 0) ? 1 : 0; //5, add a line for remainders
System.out.print("3");
System.out.println(decimals > 0 ? "." : "");
for (int line = 0; line < lines; line++) {
int offset = line * DIGITS_PER_LINE; //line -> result, 0 -> 0, 1 -> 10, 2 -> 20, etc
int digitsLeft = decimals - offset; //0 -> 42, 1 -> 32, ... , 4 -> 2
int toPrint = digitsLeft % DIGITS_PER_LINE; //up to the requested digit amount
for (int i = 0; i < toPrint; i++) {
System.out.print(pi.charAt(offset + i));
}
System.out.println();
}
请记住,代码中的整数算术不会舍入;49/10is 4, and 49%10is 9(模或余数运算符)。
该语法boolean ? "something" : "another"称为三元语句,如果它们令人困惑,我可以在没有它们的情况下重写它。您需要处理用户输入等,但永远不要害怕解决问题。从一个可以以可变形式打印输入的 x 个数字的程序开始,然后处理输入。您可以将类似的内容放入方法中printDigitsPI(int decimals)。上面使用的大多数变量都是常量,它们不会改变,也不需要传递。
作为最后一条建议,如果您发现自己将num1, num2, num3... 写为变量,那么很可能有更好的方法来解决它。
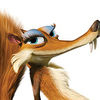
TA贡献1946条经验 获得超4个赞
下面使用 Math.PI 创建 Double PI 的字符串表示形式。然后,我们使用 String#indexOf 确定该字符串中小数点的位置。然后,我们使用 Scanner 请求我们希望看到的小数位数。假设输入是一个非负整数,我们尝试使用初始索引“.”查找字符串 PI 的子字符串。以及剩余的数字。
public static void main(String[] args) {
requestInput();
}
private static void requestInput() {
String pi = Double.toString(Math.PI);
int decimalOffset = pi.indexOf(".") + 1;
try (Scanner scanner = new Scanner(System.in)) {
System.out.println("Enter the number of decimals of PI you would like to see: ");
int decimals = scanner.nextInt();
if (decimals < 0 || decimals > pi.length() + decimalOffset) {
requestInput();
return;
}
String result = pi.substring(0, decimals + decimalOffset);
System.out.println(String.format("The result of PI with %s numbers is %s", decimals, result));
}
}
添加回答
举报