5 回答
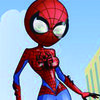
TA贡献1851条经验 获得超4个赞
这只是将 json 字符串简单地复制/粘贴到 AndroidStudio 中,它会自动分割字符串并添加转义斜杠。它看起来很糟糕,但你编写的语法完全没问题。
String jsonString = " {\n" +
" \"query\": {\n" +
" \"bool\": {\n" +
" \"must\": [\n" +
" {\"match\": \n" +
" { \"customer.partnerName\": \"Synapse\" }},\n" +
"\n" +
" {\n" +
"\"range\" : \n" +
"{\n" +
" \"customer.billing.chargeAmount\" : {\n" +
" \"gte\" : 1,\n" +
" \"lte\" : 100\n" +
" }\n" +
" }}\n" +
" ],\n" +
" \"filter\": [\n" +
" { \"match\": { \"customer.configId\": 15 }}\n" +
" ]\n" +
" }\n" +
" }\n" +
" }";
// HERE BEAUTIFIED
/*jsonString = "{\"query\":{\"bool\":{\"must\":[{\"match\":{\"customer.partnerName\":\"Synapse\"}},{\"range\":{\"customer.billing.chargeAmount\":{\"gte\":1,\"lte\":100}}}],\"filter\":[{\"match\":{\"customer.configId\":15}}]}}}";
*/
try {
JSONObject object = new JSONObject(jsonString);
// NO ERRORS, OBJECT CREATED IN MY CASE
} catch (JSONException e) {
e.printStackTrace();
}
您拥有的第二个选项是以编程方式创建对象、内部对象和数组..就像这样..
try {
JSONObject jsonObject = new JSONObject();
JSONObject query = new JSONObject();
jsonObject.put("query", query);
JSONObject bool = new JSONObject();
query.put("bool", bool);
JSONArray must = new JSONArray();
bool.put("must", must);
JSONObject matchWrap = new JSONObject();
JSONObject match = new JSONObject();
match.put("customer.partnerName", "Synapse");
matchWrap.put("match", match);
must.put(matchWrap);
JSONObject rangeWrap = new JSONObject();
JSONObject range = new JSONObject();
JSONObject customerBillingChargeAmount = new JSONObject();
customerBillingChargeAmount.put("gte", 1);
customerBillingChargeAmount.put("lte", 100);
range.put("customer.billing.chargeAmount", customerBillingChargeAmount);
rangeWrap.put("range", range);
must.put(rangeWrap);
JSONArray filter = new JSONArray();
bool.put("filter", filter);
JSONObject match2Wrap = new JSONObject();
JSONObject match2 = new JSONObject();
match2.put("customer.configId", 15);
match2Wrap.put("match", match2);
filter.put(match2Wrap);
String jsonString2 = jsonObject.toString();
// HERE THE SAME JSON STRING AS YOUR INPUT
} catch (JSONException e) {
e.printStackTrace();
}
当删除空格、制表符、换行符等时,这会产生与输入字符串相同的结果。
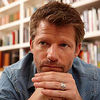
TA贡献1856条经验 获得超17个赞
我认为你正在寻找的是 json 解析。这是通过以下方式完成的:
JsonParser parser = new JsonParser();
JsonObject object = (JsonObject) parser.parse(jsonData); //Insert json string data
//Do other stuff
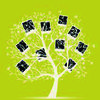
TA贡献1891条经验 获得超3个赞
所以,我想说你应该使用 JsonPath lib 来做到这一点。
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<version>2.4.0</version>
</dependency>
使用示例
import com.jayway.jsonpath.DocumentContext;
import com.jayway.jsonpath.JsonPath;
...
public void handle(...) {
...
DocumentContext jsonContext = JsonPath.parse(responseBody);
JSONArray jsonPathPreviousUrl = jsonContext.read("$..previous")
...
这将快速有效地解析您的 json。
{
"feed": {
"data": [
{
"created_time": "2017-12-12T01:24:21+0000",
"message": "This picture of my grandson with Santa",
"id": ""
},
{
"created_time": "",
"message": "",
"id": ""
},
{
"created_time": "",
"message": "",
"id": ""
}
],
"paging": {
"previous": "https://facebook/v3.2/{your-user-id}/feed?format=json&limit=3&since=1542820440",
"next": "https://facebook/v3.2/{your-user-id}/feed?format=json&limit=3&until=1542583212&"
}
},
"id": "{your-user-id}"
}
}
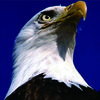
TA贡献1893条经验 获得超10个赞
<script>
var txt = '{"query": {"bool": {"must": [{"match": { "customer.partnerName": "Synapse" }},{"range" : { "customer.billing.chargeAmount" : { "gte" : 1, "lte" : 100 } }}],"filter": [{ "match": { "customer.configId": 15 }}]}}}'
var obj = JSON.parse(txt);
debugger;
document.getElementById("demo").innerHTML = obj.query;
</script>
添加回答
举报