2 回答
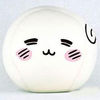
TA贡献1821条经验 获得超6个赞
您可以使用 Json.Net 的LINQ-to-JSON API 执行以下操作:
var namesToKeep = new string[] { "ID", "Code", "OrderDetails", "ItemID", "Quantity" };
var jArray = JArray.Parse(jsonString);
foreach (var prop in jArray.Descendants().OfType<JProperty>().ToList())
{
if (!namesToKeep.Contains(prop.Name))
prop.Remove();
}
jsonString = jArray.ToString();
小提琴: https: //dotnetfiddle.net/Pj9Wsu
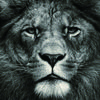
TA贡献1869条经验 获得超4个赞
为了从序列化中排除属性,您需要使用[JsonIgnore]attribute 来标记属性。
像这样的东西:
public class OrderDetail
{
public int ID { get; set; }
public int OrderID { get; set; }
public int ItemID { get; set; }
[JsonIgnore]
public double Quantity { get; set; }
[JsonIgnore]
public double ConfirmQuantity { get; set; }
[JsonIgnore]
public DateTime ConfirmDate { get; set; }
[JsonIgnore]
public DateTime Deadline { get; set; }
[JsonIgnore]
public bool IsCanceled { get; set; }
[JsonIgnore]
public int PersonnelID { get; set; }
[JsonIgnore]
public bool IsConfirmed { get; set; }
}
public class RootObject
{
public int ID { get; set; }
public string Code { get; set; }
[JsonIgnore]
public int SupplierID { get; set; }
[JsonIgnore]
public DateTime Date { get; set; }
public List<OrderDetail> OrderDetails { get; set; }
}
但我实际上建议您将原始集合投影到具有属性子集的对象集合中。
最简单的方法是仅使用要序列化的属性定义新类,并将其从一个类映射到另一个类(使用普通 LINQSelect或某种映射器):
public class OrderDetailModel
{
public int ID { get; set; }
public int OrderID { get; set; }
public int ItemID { get; set; }
}
public class RootObjectModel
{
public int ID { get; set; }
public string Code { get; set; }
public List<OrderDetailModel> OrderDetails { get; set; }
}
- 2 回答
- 0 关注
- 109 浏览
添加回答
举报