2 回答
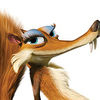
TA贡献1859条经验 获得超6个赞
可能有一百万种方法可以做到这一点,但最终您将需要一些能够过滤值和该值的路径的递归方法。为此,以下是这百万种方法中的一种。
它使用预定义的迭代器:
回调过滤器迭代器
递归迭代器迭代器
递归数组迭代器
与自定义PathAsKeyDecorator
迭代器结合使用。
<?php
declare(strict_types=1);
final class PathAsKeyDecorator implements \Iterator
{
private RecursiveIteratorIterator $inner;
public function __construct(RecursiveIteratorIterator $inner)
{
$this->inner = $inner;
}
public function current()
{
return $this->inner->current();
}
public function next(): void
{
$this->inner->next();
}
public function key()
{
$path = [];
for ($i = 0, $depth = $this->inner->getDepth(); $i <= $depth; $i++) {
$path[] = $this->inner->getSubIterator($i)->key();
}
return $path;
}
public function valid(): bool
{
return $this->inner->valid();
}
public function rewind(): void
{
$this->inner->rewind();
}
}
$input = [
'steve' => [
'id' => [
'#text' => 1,
],
],
'albert' => [
'id' => [
'#text' => 2,
],
],
'john' => [
'profil' => [
'id' => [
'#text' => 3,
],
],
],
];
// this is the filter function that should be customized given your requirements
// or create a factory function which produces these types of filter functions
$filter = static function ($current, array $path): bool {
// with help from the PathAsKeyDecorator
// we can decide on the path to the current value
return ['id', '#text'] === array_slice($path, -2)
// and the current value
&& 2 === $current;
};
// configure the iterator
$it = new CallbackFilterIterator(
new PathAsKeyDecorator(new RecursiveIteratorIterator(new RecursiveArrayIterator($input))),
$filter,
);
// traverse the iterator
foreach ($it as $path => $val) {
print_r([
'path' => $path,
'val' => $val
]);
}
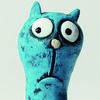
TA贡献1799条经验 获得超9个赞
完整编辑阵列结构变化的原因。json_encode 用于将数组更改为字符串。仅当每个数组切片中有一个 id 和一个伪值时,它才起作用。
$data = [
'steve' => [
'id' => [
'#text' => 1,
],
'pseudo' => [
'#text' => 'LOL'
],
],
'albert' => [
'id' => [
'#text' => 2,
],
'pseudo' => [
'#text' => 'KILLER'
],
],
'john' => [
'id' => [
'#text' => 3,
],
'pseudo' => [
'#text' => 'NOOBS'
],
],
];
$data = json_encode($data, JSON_NUMERIC_CHECK);
preg_match_all('~"id":{"#text":([^{]*)}~i', $data, $ids);
preg_match_all('~"pseudo":{"#text":"([^{]*)"}~i', $data, $pseudos);
$lnCounter = 0;
$laResult = array();
foreach($ids[1] as $lnId) {
$laResult[$lnId] = $pseudos[1][$lnCounter];
$lnCounter++;
}
echo $laResult[2];
- 2 回答
- 0 关注
- 85 浏览
添加回答
举报