2 回答
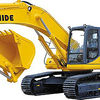
TA贡献1852条经验 获得超7个赞
如果类别(例如:代理、客人)是固定的并且您知道所有可能的组合是什么,您可以像这样进行选择
var $main = $('#main');
// filter all the class that are not in the main
var aClasses = ['agent', 'guest'].filter(function(cl) {
return !$main.hasClass(cl)
});
// build the selector
var selector = ':not(.hidden)';
aClasses.forEach(function(cl) {
selector += ':not([data-access="' + cl + '"])'
})
$('div.area' + selector).each(function(i, el) {
$('div.section' + selector, el).each(function(_i, _el) {
$('div[data-type]' + selector, _el).each(function(__i, __el) {
// you are inside the visible 'div[data-type]' here; do your stuff
});
});
});
或者,像这样一下子做:
$(
'div.area' + selector
+ ' div.section' + selector
+ ' div[data-type]' + selector
).each( function(i, el) {
// your stuff
}
或者,如果您确实不想根据具有类(例如:代理、访客)的主 div 进行选择并检查完全相同的内容,您可以尝试
var $main = $('#main');
// get the main div's class
var sClass = (['agent', 'guest'].filter(function(cl) {
return $main.hasClass(cl)
})[0];
// make the two selector combination
var s1 = ':not(.hidden):not([data-access])';
s2 = '[data-access="' + sClass + '"]:not(.hidden)';
$('div.area' + s1 + ',div.area' + s2).each(function(i, el) {
$('div.section' + s1 + ',div.section' + s2, el).each(function(_i, _el) {
$('div[data-type]' + s1 + ',div[data-type]' + s2, el).each(function(__i, __el) {
// your stuff
});
});
});
但在这里,要一次性写出所有内容,您必须使用 8 种不同的组合
例如:
// area-s1 sect-s1 div-s1,
// area-s1 sect-s1 div-s2,
// area-s1 sect-s2 div-s1,
// area-s1 sect-s2 div-s2,
// area-s2 sect-s1 div-s1,
// area-s2 sect-s1 div-s2,
// area-s2 sect-s2 div-s1,
// area-s2 sect-s2 div-s2,
// ie:
$(
'div.area' + s1 + ' div.section' + s1 + ' div[data-type]' + s1
+ ',div.area' + s1 + ' div.section' + s1 + ' div[data-type]' + s2
+ ',div.area' + s1 + ' div.section' + s2 + ' div[data-type]' + s1
+ ',div.area' + s1 + ' div.section' + s2 + ' div[data-type]' + s2
+ ',div.area' + s2 + ' div.section' + s1 + ' div[data-type]' + s1
+ ',div.area' + s2 + ' div.section' + s1 + ' div[data-type]' + s2
+ ',div.area' + s2 + ' div.section' + s2 + ' div[data-type]' + s1
+ ',div.area' + s2 + ' div.section' + s2 + ' div[data-type]' + s2
).each(function(i, el) {
// your stuff
})
所以最好使用嵌套循环本身。
例子
// assume the classes are (agent, guest) and main div is having class 'agent' then
/* First approch */
$('div.area:not(.hidden):not([data-access="guest"] div.section:not(.hidden):not([data-access="guest"] div[data-type]:not(.hidden):not([data-access="guest"]').each(function(index, elem) {
//your stuff
})
// using nested loops
$('div.area:not(.hidden):not([data-access="guest"]').each(function(i, el) {
$('div.section:not(.hidden):not([data-access="guest"'], el).each(function(_i, _el) {
$('div[data-type]:not(.hidden):not([data-access="guest"'], _el).each(function(__i, __el) {
// you are inside the visible 'div[data-type]' here; do your stuff
});
});
});
/* Second approch */
$(
'div.area:not(.hidden):not([data-access]) div.section:not(.hidden):not([data-access]) div[data-type]:not(.hidden):not([data-access]), '
+ 'div.area:not(.hidden):not([data-access]) div.section:not(.hidden):not([data-access]) div[data-type][data-access="agent"]:not(.hidden), '
+ ...
).each(function(i, el) {
//your stuff
})
// using nested loops
$('div.area:not(.hidden):not([data-access]), div.area[data-access="agent"]:not(.hidden)').each(function(i, el) {
$('div.section:not(.hidden):not([data-access]), div.section[data-access="agent"]:not(.hidden)', el).each(function(_i, _el) {
$('div[data-type]:not(.hidden):not([data-access]), div[data-type][data-access="agent"]:not(.hidden)', _el).each(function(__i, __el) {
// your stuff
});
});
});
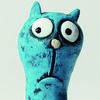
TA贡献1802条经验 获得超5个赞
jQuery 的:visible伪类将仅过滤[data-type]用户可见的元素。
因此,根据您的描述,在选择器上使用它应该足够了:
<script>
$(function () {
function fetchFormData() {
var result = [];
$('[data-name=holder] [data-type]:visible').each(function (idx, elem) {
var $elem = $(elem);
var type = $elem.data('type');
var label, value;
// specific case: radio input
if (type === 'radio') {
label = $elem.find('legend').text();
value = $elem.find('input[type=radio]:checked').val();
result.push({label: label, value: value});
// done with this item, skip to next one
return true; // continue;
}
// generic case, works with most inputs
label = $elem.find('label').text();
value = $elem.find('input').val();
result.push({label: label, value: value});
});
return result;
}
// add this to an event handler
console.log(fetchFormData());
});
</script>
添加回答
举报