2 回答
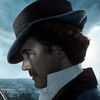
TA贡献1772条经验 获得超6个赞
所以让我们用最少的代码工作:
const goal = '4002,4003,4004,4005,4006,4007';
const test ='3500,3700,4002,4006,4007,4005,4004,4003';
如果每个测试数字都包含在目标内,您需要一个返回 true 的方法。
function inside(a, b) {
//array of numbers
const aV = a.split(',').map( e => +e);
const bV = b.split(',').map( e => +e);
//every b must be in a
return bV.every( v => aV.indexOf(v) !== -1 );
}
const goal = '1,2,3';
const test ='1,2';
function inside(b, a) {
//array of numbers
const aV = a.split(',').map( e => +e);
const bV = b.split(',').map( e => +e);
//every b must be in a
return bV.every( v => aV.indexOf(v) !== -1 );
}
console.log('Is', test);
console.log('inside', goal);
console.log('=>', inside(test, goal) );
编辑
这是一个逐步解决方案您需要一个接受两个字符串并返回一个布尔值的函数。
function inside(a,b) {
return true;
}
使用“数组作为逗号分隔的字符串”是痛苦的......让我们将字符串转换为数组,用“,”分割
function inside(b, a) {
//array of numbers
const aV = a.split(',');
const bV = b.split(',');
return true
}
然后我从字符串数组更改为数字数组,.map( e => +e)这完全没用哈哈...抱歉...所以让我们忘记这一点
好的,我们要确保 Bv 中的每个值都在 aV 中。因此,对于 Bv 中的给定值(如 x),我们想要测试 x 是否在 aV 中。让我们检查 aV 中 x 的索引:aV.indexOf(x) 如果是-1,则意味着not present......
太棒了,让我们为每一个bV !
function inside(b, a) {
//array of numbers
const aV = a.split(',');
const bV = b.split(',');
for( let i = 0; i < bV.length; i++) {
const x = bV[i];
const index = aV.indexOf(x);
if ( index === -1 ) {
//Not present, we stop here
return false;
}
}
//So we check for every Bv and none were absent, let's return true
return true;
}
好的,它可以工作,但是......我不喜欢使用for数组,让我们这样做forEach
function inside(b, a) {
//array of numbers
const aV = a.split(',');
const bV = b.split(',');
//Let's define everything is ok
let result = true;
bV.forEach( x => {
if ( aV.indexOf(x) === -1 )
result = false;
})
return result;
}
很好,但这正是该方法的重点every!
function inside(b, a) {
//array of numbers
const aV = a.split(',');
const bV = b.split(',');
return bV.every( x => {
if ( aV.indexOf(x) === -1 )
result = false;
return true;
})
}
让我们简短地说
function inside(b, a) {
//array of numbers
const aV = a.split(',').map( e => +e);
const bV = b.split(',').map( e => +e);
//every b must be in a
return bV.every( v => aV.indexOf(v) !== -1 );
}
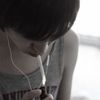
TA贡献1797条经验 获得超6个赞
您可以使用一些内置的 javascript 函数来完成此操作。
首先拆分值,将它们存储在数组中并对值进行排序。您可以比较该数组的元素是否等于源数组。
我做了一个jsfiddle来解释它。 https://jsfiddle.net/3cLa5614/
function checkValues(input) {
const sources = document.getElementById('source').value.split(',').sort();
const values = input.value.split(',').sort();
let comparator = sources.splice(0,values.length);
return JSON.stringify(comparator)==JSON.stringify(values)
}
添加回答
举报