4 回答
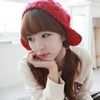
TA贡献1827条经验 获得超9个赞
您可以使用多态性在运行时调用正确实例的方法。为此,您可以创建一个Shape具有该printArea()方法的接口。该方法将由具体类实现Rectangle,并Circle定义其特定行为:
interface Shape {
void printArea();
}
class Rectangle implements Shape {
private int width;
private int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public int getArea() {
return width * height;
}
public void printArea() {
System.out.println(this.getArea());
}
}
class Circle implements Shape {
private int radius;
public Circle(int radius) {
this.radius = radius;
}
public int getArea() {
return radius * radius * 3;
}
public void printArea() {
System.out.println(this.getArea());
}
}
然后会有一个调度程序类Printer,它接受一个形状对象并调用printArea()它的方法:
class Printer {
public void printArea(Shape shape) {
shape.printArea();
}
}
然后你可以这样做来使用 Java 中的动态方法分派概念:
public static void main(String[] args) {
Shape rectangle = new Rectangle(10, 20);
rectangle.printArea();
Shape circle = new Circle(20);
circle.printArea();
Printer printer = new Printer();
printer.printArea(circle);
printer.printArea(rectangle);
}
编辑
如果您无法在类中添加新方法,那么您可以在Java 8 引入的接口default中添加方法:Shape
interface Shape {
int getArea();
default void printArea(){
System.out.println(this.getArea());
}
}
现在假设您需要一个Square实现,并且已定义getArea,那么您不再需要printArea()在类中定义方法Square:
class Square implements Shape{
private int side;
public Square(int side) {
this.side = side;
}
public int getArea() {
return side * side;
}
}
这样,当您Shape在现有类中实现新接口时,就不会破坏现有的实现。
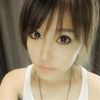
TA贡献1775条经验 获得超11个赞
您需要编写一个带有 getArea() 方法的接口。并且您应该让 Rectangle 和 Circle 实现该接口。
public interface Shape {
int getArea();
}
public class Rectangle implements Shape {
private int width;
private int height;
public Rectangle(int width,int height) {
this.width = width;
this.height = height;
}
@Override
public int getArea() {
return width * height;
}
}
public class Circle implements Shape {
private int radius;
public Circle(int radius) {
this.radius = radius;
}
@Override
public int getArea() {
return radius * radius * 3;
}
}
和..
public void PrintArea(Object object) {
if(object instanceof Shape)
System.out.print((Shape)object.getArea());
}
//or..
public void PrintArea(Shape object) {
object.getArea();
}
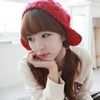
TA贡献1876条经验 获得超7个赞
您可以在 printArea 方法中使用反射,但不推荐这样做。使用接口或从抽象基类扩展
一种方法如下。
public void PrintArea(Object object) throws Exception {
Method method = object.getClass().getMethod("getArea");
System.out.println(method.invoke(object));
}
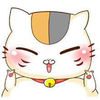
TA贡献1864条经验 获得超2个赞
假设您有两个类:矩形和圆形。
这两个类,你不能改变,所以你不能让它们实现一个接口。
但是,您可以在本地扩展它们,并让这些子类实现接口。
public interface Shape {
int getArea();
}
并且,您创建两个新类:
public class MyRectangle extends Rectangle implements Shape {
}
public class MyCircle extends Rectangle implements Shape {
}
由于这些类继承了其父类的成员,并且这些类已经提供了 getArea() 的实现,因此您所需要做的就是这些。
然后,将 printArea 方法更改为:
public void printArea(Shape myShape) {
System.out.println(myShape.getArea());
}
为了使其工作,您需要使用实例化新类而不是旧类,或者需要添加映射器,这可能类似于:
public static Shape createMyShapes(Object o) throws TechnicalException {
if ( !(o instanceof Circle || o instanceof Rectangle) )
throw new TechnicalException("Wrong type");
if ( o instanceof Circle )
return new MyCircle(o);
return new MyRectangle(o);
}
并在必要时使用它。
添加回答
举报