3 回答
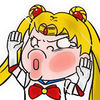
TA贡献1856条经验 获得超11个赞
首先,您应该将验证设置为同时在服务器端和客户端工作。客户端,主要是为了用户体验,因为精通开发的人总是可以绕过浏览器中的任何内容。但是,根据您的声明,您希望有一条漂亮的用户消息并显示该消息,无论客户端是什么,这将帮助您的用户更好地了解如何使用您的表单。服务器端验证也应该到位,以防止错误数据保存在数据库中。
简而言之,您需要他们携手合作。您不需要任何特殊的 js 库来进行最基本的验证。HTML5 将为您提供显示许多需求所需的内容,而无需额外的 JavaScript。但是,如果您不喜欢 HTML5 验证的外观,则需要使用某种 JavaScript。但是,我总是会从使用它开始,因为它将帮助您的应用程序更轻松地满足可访问性标准,并且从那里,您可以通过在其上添加更精美的基于 javascript 的东西来进行迭代,使其看起来更好。
该片段将有一个警告叠加层,该叠加层不会出现在您的网站上。
<form action="">
<label for="pwd">Password:</label>
<input type="password" id="pwd" name="pwd"
pattern="(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{8,}"
title="Must contain at least one number and one uppercase and lowercase letter, and at least 8 or more characters">
<input type="submit">
</form>
现在,在后端,您应该在处理表单的代码中创建一个函数,以在将其与其他数据库规则一起保存到数据库之前重新检查验证。如果由于用户或某些错误以某种方式允许绕过验证而导致此验证失败,您将在表单响应中发回一条失败消息,前端将在尝试保存到数据库失败后捕获并显示该消息。要回答具体如何进行,需要更多有关如何设置应用程序的信息。
您还可以在数据库中创建一个表来存储验证规则,并可以通过某种 API 将这些规则传递到前端,以便前端和后端函数可以共享相同的规则,而不必硬编码相同的正则表达式或无论你正在处理什么规则。换句话说,这两个验证的单一事实点——用户体验检查和数据完整性检查。
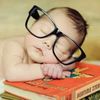
TA贡献1757条经验 获得超7个赞
我正在使用验证密码来检测检测到的弱密码或强密码:
我有两个例子:
This is Pure Javascript
var code = document.getElementById("password");
var strengthbar = document.getElementById("meter");
var display = document.getElementsByClassName("textbox")[0];
code.addEventListener("keyup", function() {
checkpassword(code.value);
});
function checkpassword(password) {
var strength = 0;
if (password.match(/[a-z]+/)) {
strength += 1;
}
if (password.match(/[A-Z]+/)) {
strength += 1;
}
if (password.match(/[0-9]+/)) {
strength += 1;
}
if (password.match(/[$@#&!]+/)) {
strength += 1;
}
if (password.length < 6) {
display.innerHTML = "minimum number of characters is 6";
}
if (password.length > 12) {
display.innerHTML = "maximum number of characters is 12";
}
switch (strength) {
case 0:
strengthbar.value = 0;
break;
case 1:
strengthbar.value = 25;
break;
case 2:
strengthbar.value = 50;
break;
case 3:
strengthbar.value = 75;
break;
case 4:
strengthbar.value = 100;
break;
}
}
<body>
<form class="center-block">
<input type="text" id="password" autocomplete="off" class="form-control input-lg">
<progress max="100" value="0" id="meter"></progress>
</form>
<div class="textbox text-center"> password </div>
</body>
这是 jQuery
function ValidatePassword() {
/*Array of rules and the information target*/
var rules = [{
Pattern: "[A-Z]",
Target: "UpperCase"
},
{
Pattern: "[a-z]",
Target: "LowerCase"
},
{
Pattern: "[0-9]",
Target: "Numbers"
},
{
Pattern: "[!@@#$%^&*]",
Target: "Symbols"
}
];
//Just grab the password once
var password = $(this).val();
/*Length Check, add and remove class could be chained*/
/*I've left them seperate here so you can see what is going on */
/*Note the Ternary operators ? : to select the classes*/
$("#Length").removeClass(password.length > 6 ? "glyphicon-remove" : "glyphicon-ok");
$("#Length").addClass(password.length > 6 ? "glyphicon-ok" : "glyphicon-remove");
/*Iterate our remaining rules. The logic is the same as for Length*/
for (var i = 0; i < rules.length; i++) {
$("#" + rules[i].Target).removeClass(new RegExp(rules[i].Pattern).test(password) ? "glyphicon-remove" : "glyphicon-ok");
$("#" + rules[i].Target).addClass(new RegExp(rules[i].Pattern).test(password) ? "glyphicon-ok" : "glyphicon-remove");
}
}
/*Bind our event to key up for the field. It doesn't matter if it's delete or not*/
$(document).ready(function() {
$("#NewPassword").on('keyup', ValidatePassword)
});
.glyphicon-remove {
color: red;
}
.glyphicon-ok {
color: green;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<div class="form-group has-feedback">
<input class="form-control" id="NewPassword" placeholder="New Password" type="password">
<span class="glyphicon glyphicon-lock form-control-feedback"></span>
</div>
<div id="Length" class="input glyphicon-remove">Must be at least 7 charcters</div>
<div id="UpperCase" class="glyphicon glyphicon-remove">Must have atleast 1 upper case character</div>
<div id="LowerCase" class="glyphicon glyphicon-remove">Must have atleast 1 lower case character</div>
<div id="Numbers" class="glyphicon glyphicon-remove">Must have atleast 1 numeric character</div>
<div id="Symbols" class="glyphicon glyphicon-remove">Must have atleast 1 special character</div>
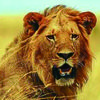
TA贡献1803条经验 获得超3个赞
您可以在 javascript 代码中使用 jquery.validate,
<script>
$("#form1").validate({
rules:{
password:{
required:true,
minlength:8
}
},
messages:{
password:{
required:"Password is required",
minlength:"Passowrd must be atleast 8 characters long"
}
}
});
</script>
密码是输入字段中的名称属性,对于数据库中的图片,您可以使用 varbinary(max)
添加回答
举报