1 回答
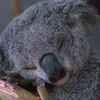
TA贡献1818条经验 获得超8个赞
问题
React.createRef
实际上只在基于类的组件中有效。如果在功能组件主体中使用,则将在每个渲染周期重新创建引用。不要使用 DOM 查询选择器将
onClick
侦听器附加到 DOM 元素。这些存在于外部反应,您需要记住清理它们(即删除它们),这样就不会出现内存泄漏。使用 React 的onClick
prop。映射后,您将相同的
selectedElements
引用附加到每个元素,因此最后一组是您的 UI 获取的元素。
解决方案
在功能组件主体中使用
React.useRef
来存储反应引用数组,以附加到要滚动到视图中的每个元素。scrollSmoothHandler
将直接附加到每个span
的onClick
支柱上。将 1. 中创建的引用数组中的每个引用附加到要滚动到的每个映射字段集。
代码
import React, { createRef, useRef } from "react";
import { render } from "react-dom";
const App = () => {
const selectedElements = [
"Item One",
"Item Two",
"Item Three",
"Item Four",
"Item Five"
];
// React ref to store array of refs
const scrollRefs = useRef([]);
// Populate scrollable refs, only create them once
// if the selectedElements array length is expected to change there is a workaround
scrollRefs.current = [...Array(selectedElements.length).keys()].map(
(_, i) => scrollRefs.current[i] ?? createRef()
);
// Curried handler to take index and return click handler
const scrollSmoothHandler = (index) => () => {
scrollRefs.current[index].current.scrollIntoView({ behavior: "smooth" });
};
return (
<div>
<div id="container">
<div className="_2iA8p44d0WZ">
{selectedElements.map((el, i) => (
<span
className="chip _7ahQImy"
onClick={scrollSmoothHandler(i)} // <-- pass index to curried handler
>
{el}
</span>
))}
<input
type="text"
className="searchBox"
id="search_input"
placeholder="Select"
autoComplete="off"
value=""
/>
</div>
</div>
<div>
{selectedElements.map((item, i) => (
<div
key={i}
className="selected-element"
ref={scrollRefs.current[i]} // <-- pass scroll ref @ index i
>
<fieldset>
<legend>{item}</legend>
</fieldset>
</div>
))}
</div>
</div>
);
};
解决方案#2
由于您无法更新div
with中的任何元素id="container"
,并且所有onClick
处理程序都需要通过查询 DOM 来附加,因此您仍然可以使用柯里化scrollSmoothHandler
回调并将索引包含在范围内。您需要一个钩子来在初始渲染后useEffect
查询 DOM ,以便安装跨度,并需要一个钩子来存储“已加载”状态。该状态是触发重新渲染并重新包含在回调中所必需的。useState
scrollRefs
scrollSmoothHandler
const App = () => {
const selectedElements = [
"Item One",
"Item Two",
"Item Three",
"Item Four",
"Item Five"
];
const [loaded, setLoaded] = useState(false);
const scrollRefs = useRef([]);
const scrollSmoothHandler = (index) => () => {
scrollRefs.current[index].current.scrollIntoView({ behavior: "smooth" });
};
useEffect(() => {
const chipsArray = document.querySelectorAll("#container > div > .chip");
if (!loaded) {
scrollRefs.current = [...Array(chipsArray.length).keys()].map(
(_, i) => scrollRefs.current[i] ?? createRef()
);
chipsArray.forEach((elem, index) => {
elem.addEventListener("click", scrollSmoothHandler(index));
});
setLoaded(true);
}
}, [loaded]);
return (
<div>
<div id="container">
<div className="_2iA8p44d0WZ">
<span className="chip _7ahQImy">Item One</span>
<span className="chip _7ahQImy">Item Two</span>
<span className="chip _7ahQImy">Item Three</span>
<span className="chip _7ahQImy">Item Four</span>
<span className="chip _7ahQImy">Item Five</span>
<input
type="text"
className="searchBox"
id="search_input"
placeholder="Select"
autoComplete="off"
value=""
/>
</div>
</div>
<div>
{selectedElements.map((item, i) => (
<div key={i} className="selected-element" ref={scrollRefs.current[i]}>
<fieldset>
<legend>{item}</legend>
</fieldset>
</div>
))}
</div>
</div>
);
};
添加回答
举报