2 回答
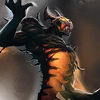
TA贡献1836条经验 获得超4个赞
进行了一些修改,以适应问题中的要求,并在找到目标之一时中断 - 从这里:
from collections import defaultdict
# This class represents a directed graph using
# adjacency list representation
class Graph:
# Constructor
def __init__(self):
# default dictionary to store graph
self.graph = defaultdict(list)
# function to add an edge to graph
def addEdge(self, u, v):
self.graph[u].append(v)
# A function used by DFS
def DFSUtil(self, v, visited, goals):
# Mark the current node as visited
# and print it
print(" ")
visited[v] = True
for goal in goals:
if v == goal:
print(f"found {v} - finish!")
return
print(v, end = ' ')
# Recur for all the vertices
# adjacent to this vertex
for i in self.graph[v]:
if visited[i] == False:
self.DFSUtil(i, visited, goals)
# The function to do DFS traversal. It uses
# recursive DFSUtil()
def DFS(self, v, goals):
# Mark all the vertices as not visited
visited = [False] * (max(self.graph)+1)
# Call the recursive helper function
# to print DFS traversal
self.DFSUtil(v, visited, goals)
# Driver code
# Create a graph given
# in the above diagram
g = Graph()
g.addEdge(0, 1)
g.addEdge(0, 2)
g.addEdge(1, 2)
g.addEdge(2, 0)
g.addEdge(2, 3)
g.addEdge(3, 3)
print("Following is DFS from (starting from vertex 2)")
g.DFS(2, [1,4])
输出:
Following is DFS from (starting from vertex 2)
2
0
found 1 - finish!
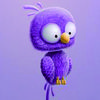
TA贡献1796条经验 获得超4个赞
# Using a Python dictionary to act as an adjacency list
graph = {
'A' : ['B','C'],
'B' : ['D', 'E'],
'C' : ['F'],
'D' : [],
'E' : ['F'],
'F' : []
}
visited = set() # Set to keep track of visited nodes.
def dfs(visited, graph, node):
if node not in visited:
print (node)
visited.add(node)
for neighbour in graph[node]:
dfs(visited, graph, neighbour)
# Driver Code
dfs(visited, graph, 'A')
这就是 dfs 的工作原理
添加回答
举报