3 回答
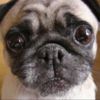
TA贡献1735条经验 获得超5个赞
您需要创建一个频道,例如
hashMap[key] = make(chan int)
由于您没有从通道中读取数据,因此需要缓冲通道才能使其工作:
key := "xyz" hashMap[key] = make(chan int, 5)
尝试以下代码:
func put(hashMap map[string](chan int), key string, value int, wg *sync.WaitGroup) {
hashMap[key] <- value
fmt.Printf("PUT sent %d\n", value)
wg.Done()
}
func main() {
var wg sync.WaitGroup
hashMap := map[string]chan int{}
key := "xyz"
hashMap[key] = make(chan int, 5)
for i := 0; i < 5; i++ {
wg.Add(1)
go put(hashMap, key, 100, &wg)
}
wg.Wait()
}
输出:
PUT sent 100
PUT sent 100
PUT sent 100
PUT sent 100
PUT sent 100
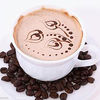
TA贡献1878条经验 获得超4个赞
我解决该问题的解决方案是:
// PUT function
func put(hashMap map[string](chan int), key string, value int, wg *sync.WaitGroup) {
defer wg.Done()
fmt.Printf("this is getting printed")
hashMap[key] <- value // <-- nil problem
fmt.Printf("this is not getting printed")
fmt.Printf("PUT sent %d\n", value)
}
在函数的这行代码中hashMap[key] <- value,put它不能接受,value因为它chan int是nil在参数中定义的put (hashMap map[string](chan int)。
// PUT function
func put(hashMap map[string](chan int), cval chan int, key string, value int, wg *sync.WaitGroup) {
defer wg.Done()
fmt.Println("this is getting printed")
cval <- value // put the value in chan int (cval) which is initialized
hashMap[key] = cval // set the cval(chan int) to hashMap with key
fmt.Println("this is not getting printed")
fmt.Printf("PUT sent %s %d\n", key, value)
}
func main() {
var value int
wg := &sync.WaitGroup{}
cval := make(chan int,100)
hashMap := make(map[string](chan int), 100)
value = 100
for i := 0; i < 5; i++ {
wg.Add(1)
go put(hashMap, cval, fmt.Sprintf("key%d",i), value, wg)
}
wg.Wait()
/* uncomment to test cval
close(cval)
fmt.Println("Result:",<-hashMap["key2"])
fmt.Println("Result:",<-hashMap["key1"])
cval <- 88 // cannot send value to a close channel
hashMap["key34"] = cval
fmt.Println("Result:",<-hashMap["key1"])
*/
}
在我的代码示例中。我将cval缓冲通道 100 初始化为相同大小,hashMap并将 cval 作为put函数中的值传递。您只能关闭cval而不是 hashMap 本身。
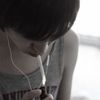
TA贡献1797条经验 获得超6个赞
另外,您的代码可以简化为这样。为什么要传递不必要的参数!一项额外的修改是我采用了不同的值,以使您更清楚地理解这个概念。
package main
import (
"log"
"sync"
)
func put(hash chan int, wg *sync.WaitGroup) {
defer wg.Done()
log.Println("Put sent: ", <-hash)
}
func main() {
hashMap := map[string]chan int{}
key := "xyz"
var wg sync.WaitGroup
hashMap[key] = make(chan int, 5)
for i := 0; i < 5; i++ {
value := i
wg.Add(1)
go func(val int) {
hashMap[key] <- val
put(hashMap[key], &wg)
}(value)
}
wg.Wait()
}
- 3 回答
- 0 关注
- 161 浏览
添加回答
举报