3 回答
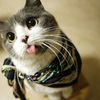
TA贡献1827条经验 获得超7个赞
如果您不想使用该库,您可以像这样实现健康检查:
import (
"google.golang.org/grpc/health"
"google.golang.org/grpc/health/grpc_health_v1"
)
grpcServer := grpc.NewServer()
grpc_health_v1.RegisterHealthServer(grpcServer, health.NewServer())
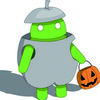
TA贡献1779条经验 获得超6个赞
您现在应该实现该逻辑,并在您的服务集中注册该服务。
自述文件建议手动将服务注册到运行状况检查服务中,以便您可以将服务列表作为参数传递来创建运行状况检查服务。
我建议为您的服务定义一个接口,以便健康检查服务可以以相同的方式处理所有这些服务:
type HealthMeter interface {
GetHealth() mysample.HealthCheckResponse_ServingStatus
WatchHealth() <- chan mysample.HealthCheckResponse_ServingStatus
}
// implement service "A" and "B" as both interfaces (gRPC server AND HealthMeter)
grpcServer := grpc.NewServer()
srvA := servers.NewServiceA()
srvB := servers.NewServiceB()
healthSrv := servers.NewHealthCheckServer(map[string]servers.HealthMeter{
"serviceA": srvA,
"serviceB": srvB,
})
mysample.RegisterServiceAServer(grpcServer, srvA)
mysample.RegisterServiceBServer(grpcServer, srvB)
mysample.RegisterHealthServer(grpcServer, healthSrv)
我希望它可以帮助你。
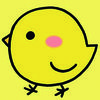
TA贡献1911条经验 获得超7个赞
在 grpc 包中实现健康检查示例的最简单方法
/*
*
* Copyright 2020 gRPC authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
// Binary server is an example server.
package main
import (
"context"
"flag"
"fmt"
"log"
"net"
"time"
"google.golang.org/grpc"
pb "google.golang.org/grpc/examples/features/proto/echo"
"google.golang.org/grpc/health"
healthgrpc "google.golang.org/grpc/health/grpc_health_v1"
healthpb "google.golang.org/grpc/health/grpc_health_v1"
)
var (
port = flag.Int("port", 50051, "the port to serve on")
sleep = flag.Duration("sleep", time.Second*5, "duration between changes in health")
system = "" // empty string represents the health of the system
)
type echoServer struct {
pb.UnimplementedEchoServer
}
func (e *echoServer) UnaryEcho(ctx context.Context, req *pb.EchoRequest) (*pb.EchoResponse, error) {
return &pb.EchoResponse{
Message: fmt.Sprintf("hello from localhost:%d", *port),
}, nil
}
var _ pb.EchoServer = &echoServer{}
func main() {
flag.Parse()
lis, err := net.Listen("tcp", fmt.Sprintf(":%d", *port))
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
s := grpc.NewServer()
healthcheck := health.NewServer()
healthgrpc.RegisterHealthServer(s, healthcheck)
pb.RegisterEchoServer(s, &echoServer{})
go func() {
// asynchronously inspect dependencies and toggle serving status as needed
next := healthpb.HealthCheckResponse_SERVING
for {
healthcheck.SetServingStatus(system, next)
if next == healthpb.HealthCheckResponse_SERVING {
next = healthpb.HealthCheckResponse_NOT_SERVING
} else {
next = healthpb.HealthCheckResponse_SERVING
}
time.Sleep(*sleep)
}
}()
if err := s.Serve(lis); err != nil {
log.Fatalf("failed to serve: %v", err)
}
}
- 3 回答
- 0 关注
- 123 浏览
添加回答
举报