1 回答
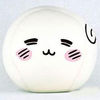
TA贡献1874条经验 获得超12个赞
该问题涉及以编程方式取消检查输入而不引发change
事件。
说明:该change
事件仅在“当用户通过单击或使用键盘提交对元素值的更改时”触发,并且“不一定在每次对元素值的更改时触发”
对于您的情况: 选中一个选项后,我们希望取消选中其他选项。我们想要添加选中选项的价格并减去未选中选项的价格。然而:
为了减去未选中的选项,我们需要触发该
change
事件。我们只想在选中某个选项时取消选中其他选项。
我们不想在取消选中某个选项时取消选中其他选项。我们只想减去从checked 变为unchecked 的选项。
我们不想减去已经未选中的选项。
所以,而不是这个:
$('.drinks').on('change', function() {
$('.drinks').not(this).prop('checked', false);
});
我们可以使用这个:
$('.drinks').on('change', function() {
if (this.checked) {
$('.drinks:checked').not(this).prop('checked', false).trigger('change');
}
}
下面,我合并了一些重复的代码,试图使事情尽可能干燥。现在,每个选项都有相同的名称“drink”,并且单个处理程序绑定到所有选项。
示范:
$(function() {
const $priceDisplay = $('#pricedisplay');
const $drinks = $('.drinks');
let totalprice = 200;
$priceDisplay.html("$" + totalprice);
$drinks.on('change', function() {
// if changed input is checked ...
if (this.checked) {
// for all checked except this, uncheck and trigger "change" event
$drinks.filter(':checked').not(this).prop('checked', false).trigger('change');
}
// add or subtract price depending on checked state
totalprice = this.checked
? totalprice + parseFloat(this.value)
: totalprice - parseFloat(this.value);
// update display
$priceDisplay.html("$" + totalprice);
});
});
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<!DOCTYPE html>
<div class="chks">
<div id="ck-button-one">
<label>
<input class="drinks" type="checkbox" name="drink" value="5">
<span>One</span>
</label>
</div>
<div id="ck-button-two">
<label>
<input class="drinks" type="checkbox" name="drink" value="10">
<span>Two</span>
</label>
</div>
<div id="ck-button-three">
<label>
<input class="drinks" type="checkbox" name="drink" value="20">
<span>Three</span>
</label>
</div>
</div>
<div class="price">Purchase Price:
<span id="pricedisplay"></span>
</div>
替代方法
不要考虑在浮动价格中添加和减去,而是考虑仅将选定的选项添加到固定基本价格中。每次饮料选项发生变化时,通过增加所选选项的基本价格来重新计算总价。
当饮料选项发生变化时,所选饮料的价格将添加到基本价格中。
如果未选择任何饮品,则基本价格不会添加任何内容。
$(function() {
const basePrice = "200";
const $priceDisplay = $('#pricedisplay');
const $drinks = $('.drinks');
$priceDisplay.html("$" + basePrice);
$drinks.on('change', function() {
let newPrice = parseFloat(basePrice);
$drinks.not(this).prop('checked', false);
$drinks.filter(':checked').each(function() {
newPrice += parseFloat(this.value);
});
$priceDisplay.html("$" + newPrice);
});
});
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<div class="chks">
<div id="ck-button-one">
<label>
<input class="drinks" type="checkbox" name="drink" value="5">
<span>One</span>
</label>
</div>
<div id="ck-button-two">
<label>
<input class="drinks" type="checkbox" name="drink" value="10">
<span>Two</span>
</label>
</div>
<div id="ck-button-three">
<label>
<input class="drinks" type="checkbox" name="drink" value="20">
<span>Three</span>
</label>
</div>
</div>
<div class="price">Purchase Price:
<span id="pricedisplay"></span>
</div>
通过稍微不同的结构,这个概念可以扩展到处理多个选项组:
$(function() {
const basePrice = "200";
const $priceDisplay = $('#pricedisplay');
const $options = $('.option');
$priceDisplay.html("$" + basePrice);
$options.on('change', function() {
// define new price from base price
let newPrice = parseFloat(basePrice);
// uncheck other options in this group
let $thisGroupOpts = $options.filter('[name=' + this.name + ']');
$thisGroupOpts.not(this).prop('checked', false);
// add prices for all checked options
$options.filter(':checked').each(function() {
newPrice += parseFloat(this.value);
});
// display total price
$priceDisplay.html("$" + newPrice);
});
});
.optionsPane {
display: flex;
flex-direction: row;
margin: 0 0 2em;
}
.optionGroup {
flex: 1 1 auto;
}
.optionGroup label {
display: block;
}
#pricedisplay {
font-weight: bold;
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<div class="optionsPane">
<div class="optionGroup">
<h2>Drinks</h2>
<label>
<input class="option" type="checkbox" name="drink" value="5">
<span>One</span>
</label>
<label>
<input class="option" type="checkbox" name="drink" value="10">
<span>Two</span>
</label>
<label>
<input class="option" type="checkbox" name="drink" value="20">
<span>Three</span>
</label>
</div>
<div class="optionGroup">
<h2>Sides</h2>
<label>
<input class="option" type="checkbox" name="side" value="3">
<span>One</span>
</label>
<label>
<input class="option" type="checkbox" name="side" value="4">
<span>Two</span>
</label>
<label>
<input class="option" type="checkbox" name="side" value="5">
<span>Three</span>
</label>
</div>
<div class="optionGroup">
<h2>Deserts</h2>
<label>
<input class="option" type="checkbox" name="desert" value="5">
<span>One</span>
</label>
<label>
<input class="option" type="checkbox" name="desert" value="7">
<span>Two</span>
</label>
<label>
<input class="option" type="checkbox" name="desert" value="10">
<span>Three</span>
</label>
</div>
</div>
<div class="price">Purchase Price:
<span id="pricedisplay"></span>
</div>
添加回答
举报