2 回答
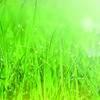
TA贡献1943条经验 获得超7个赞
JsonPath库允许您仅选择必需的字段,然后您可以使用它将Jackson
原始数据转换为POJO
类。示例解决方案如下所示:
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.type.CollectionType;
import com.jayway.jsonpath.JsonPath;
import java.io.File;
import java.util.List;
import java.util.Map;
public class JsonPathApp {
public static void main(String[] args) throws Exception {
File jsonFile = new File("./resource/test.json").getAbsoluteFile();
List<Map> nodes = JsonPath.parse(jsonFile).read("$..value[*].user.name");
ObjectMapper mapper = new ObjectMapper();
CollectionType usersType = mapper.getTypeFactory().constructCollectionType(List.class, User.class);
List<User> users = mapper.convertValue(nodes, usersType);
System.out.println(users);
}
}
class User {
@JsonProperty("first")
private String firstName;
@JsonProperty("last")
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public String toString() {
return "User{" +
"firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
'}';
}
}
上面的代码打印:
[User{firstName='x', lastName='y'}]
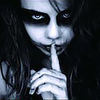
TA贡献1840条经验 获得超5个赞
另一种简单的方法是使用JSON.simple库:
JSONParser jsonParser = new JSONParser();
//Read JSON file
Object obj = jsonParser.parse(reader);
JSONObject jObj = (JSONObject) obj;
JSONObject root = (JSONObject)jObj.get("root");
JSONObject data = (JSONObject) root.get("data");
JSONArray value = (JSONArray) data.get("value");
JSONObject array = (JSONObject) value.get(0);
JSONObject user = (JSONObject) array.get("user");
JSONObject name = (JSONObject) user.get("name");
String lastName = (String) name.get("last");
String firstName = (String) name.get("first");
System.out.println(lastName + " " + firstName);
添加回答
举报