2 回答
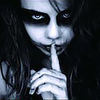
TA贡献1840条经验 获得超5个赞
您的要求不同,您不必在这里使用 componentDidMount,因为您将在用户按下搜索按钮后进行服务调用。
我已经修改了您的代码以在按下按钮时工作,并将代码传递给填充组件,该组件在 componentDidMount 和 componentDidUpdate 上调用 api,因为代码将来可能会更新
class App extends React.Component {
constructor(props) {
super(props);
this.state = { name: "", info: {}, formSubmit: false, code: "" };
this.handleInput = this.handleInput.bind(this);
this.handleFormSubmit = this.handleFormSubmit.bind(this);
}
handleInput(event) {
console.log(event.target.value);
this.setState({ name: event.target.value, formSubmit: false });
}
handleFormSubmit(event) {
event.preventDefault();
fetch(
`https://wft-geo-db.p.rapidapi.com/v1/geo/cities?namePrefix=${this.state.name}`,
{
method: "GET",
headers: {
"x-rapidapi-key":
"",
"x-rapidapi-host": "wft-geo-db.p.rapidapi.com"
}
}
)
.then((response) => response.json())
.then((data) => {
const newInfo = data.data;
const newName = newInfo[0].wikiDataId;
const newState = Object.assign({}, this.state, {
info: newInfo[0],
code: newName,
formSubmit: true
});
this.setState(newState);
console.log("The sent code " + this.state.name);
})
.catch((err) => {
console.error(err);
});
}
componentDidUpdate() {
//the value property needs to be fetched from user
}
render() {
return (
<div className="App">
<h1>
Enter the name of the city: <br />
</h1>
<form>
<label>
Enter the city name to get the population:{" "}
<input
type="text"
value={this.state.name}
onChange={this.handleInput}
/>
<button onClick={this.handleFormSubmit}>Enter</button>
</label>
</form>
{this.state.formSubmit && <Population name={this.state.code} />}
</div>
);
}
}
人口组成就像
class Population extends React.Component {
constructor(props) {
super(props);
this.state = {
info: {},
population: 0
};
this.getPopulation = this.getPopulation.bind(this);
}
getPopulation(name) {
fetch(`https://wft-geo-db.p.rapidapi.com/v1/geo/cities/${name}`, {
method: "GET",
headers: {
"x-rapidapi-key": "",
"x-rapidapi-host": "wft-geo-db.p.rapidapi.com"
}
})
.then((response) => response.json())
.then((data) => {
const newInfo = data.data;
const newPopulation = newInfo.population;
const newState = Object.assign({}, this.state, {
info: newInfo,
population: newPopulation
});
this.setState(newState);
console.log(this.state.info);
})
.catch((error) => {
console.error(error);
});
}
componentDidMount() {
if (this.props.name) {
this.getPopulation(this.props.name);
console.log("The name " + this.props.name);
}
}
componentDidUpdate() {
if (this.props.name) {
this.getPopulation(this.props.name);
console.log("The name " + this.props.name);
}
}
render() {
return <div className="App">The population is {this.state.population}</div>;
}
}
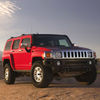
TA贡献1824条经验 获得超8个赞
一旦组件安装,就会调用 componentDidMount 函数,因此一旦组件安装,它就会执行获取操作。如果您希望在单击按钮时发生获取操作,则必须将代码放置在自定义函数中并在单击按钮时调用它。我认为您无法将任何道具传递给已安装的组件,因为您无法控制何时调用它。componentDidUpdate可能对您有用
添加回答
举报