1 回答
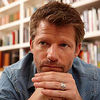
TA贡献1784条经验 获得超7个赞
让我们从火车代码开始(内联文档)
# TensorFlow and tf.keras
import tensorflow as tf
from tensorflow import keras
# Helper libraries
import numpy as np
import matplotlib.pyplot as plt
# load data
fashion_mnist = keras.datasets.fashion_mnist
(train_images, train_labels), (test_images, test_labels) = fashion_mnist.load_data()
# Text representation of labels
class_names = ['T-shirt/top', 'Trouser', 'Pullover', 'Dress', 'Coat',
'Sandal', 'Shirt', 'Sneaker', 'Bag', 'Ankle boot']
# Normalize the train and test images
train_images = train_images / 255.0
test_images = test_images / 255.0
# Define the model
model = keras.Sequential([
keras.layers.Flatten(input_shape=(28, 28)),
keras.layers.Dense(128, activation='relu'),
keras.layers.Dense(10)
])
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# train the model
model.fit(train_images, train_labels, epochs=10)
正如你所看到的,最后一层是Dense输出大小的层10。那是因为我们有10个班级。为了确定它属于哪个类别,我们只需从这 10 个类别中取出最大值并将其类别指定为预测即可。但如果我们可以将这些值更改为概率,我们还可以知道模型做出此预测的信心有多大。因此,让我们附加 softmax 层,将这 10 个输出归一化为概率。
probability_model = tf.keras.Sequential([model, tf.keras.layers.Softmax()])
predictions = probability_model.predict(test_images)
print (f"Input: {test_images.shape}, Output: {predictions.shape}")
输出:
Input: (10000, 28, 28), Output: (10000, 10)
让我们打印第 i 个测试图像的预测标签和真实标签
i = 0
print (f"Actual Label: {train_labels[i]}, Predicted Label: {np.argmax(predictions[i])}")
输出:
Actual Label: 9, Predicted Label: 9
最后让我们绘制第 i 个图像并用预测的类别及其概率对其进行标记。(内联文档)
def plot_image(i, predictions_array, true_label, img):
"""
i: render ith image
predictions_array: Probabilities of each class predicted by the model for the ith image
true_label: All the the acutal label
img: All the images
"""
# Get the true label of ith image and the ithe image itself
true_label, img = true_label[i], img[i]
plt.grid(False)
plt.xticks([])
plt.yticks([])
# Render the ith image
plt.imshow(img, cmap=plt.cm.binary)
# Get the class with the higest probability for the ith image
predicted_label = np.argmax(predictions_array)
if predicted_label == true_label:
color = 'blue'
else:
color = 'red'
plt.xlabel("{} {:2.0f}% ({})".format(class_names[predicted_label],
100*np.max(predictions_array),
class_names[true_label]),
color=color)
最后让我们调用它
plot_image(i, predictions[i], test_labels, test_images)
你的困惑是因为predictions_array
参数。请注意,这是模型对第 i 个测试数据所做的预测。它有 10 个值,每个值代表它属于相应类别的概率。
添加回答
举报