2 回答
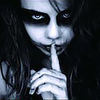
TA贡献1802条经验 获得超6个赞
以下是一些可能有帮助的事情:
ascii_lowercase模块中string是包含所有小写字母字符的预定义字符串:
>>> from string import ascii_lowercase
>>> ascii_lowercase
'abcdefghijklmnopqrstuvwxyz'
>>>
我们可以通过创建一组元音并获取元音和所有字符之间的差异来生成所有辅音的集合:
from string import ascii_lowercase as alphabet
vowels = set("aeiou")
consonants = set(alphabet) ^ vowels
print(consonants)
输出:
{'c', 's', 'q', 'm', 'g', 'd', 'y', 'l', 'b', 'k', 't', 'j', 'r', 'p', 'h', 'v', 'n', 'w', 'z', 'f', 'x'}
>>>
由于这是一个集合,因此没有内在顺序,但这并不重要。如果我们想知道给定的字符是辅音还是元音,我们只需检查相应集合的成员资格(您可以对列表执行相同的操作,但集合将是首选数据结构)。
无论您的vowelsand是否使用列表或集合consonants,您都可以通过简单地检查成员资格(检查某个字符是否在集合中)来简化代码:
if word[0] in vowels:
# The first letter is a vowel
elif word[0] in consonants:
# The first letter is a consonant
如果您事先知道word只包含小写字母字符(没有特殊符号、数字、大写字母等),那么您可以进一步简化它:
if word[0] in vowels:
# The first letter is a vowel
else:
# If it's not a vowel, it must be a consonant
然而,如果你仔细想想,你根本不需要检查第一个字母是否是元音。您已经知道您将在最终字符串的末尾添加"ay",无论第一个字母是元音还是辅音 - 因此,您实际上只需要检查第一个字母是否是辅音。
使用到目前为止的所有内容,我将得到以下伪代码:
def to_pig_latin(word):
from string import ascii_lowercase as alphabet
vowels = set("aeiou")
consonants = set(alphabet) ^ vowels
if word[0] in consonants:
# Do something
return ... + "ay"
我已重命名该 function to_pig_latin,因为首选蛇形命名法,并且在函数名称前加上前缀to表示您正在翻译/转换某些内容。vowels我还将和的创建移到consonants了函数中,只是因为没有理由将它放在函数之外,而且这样更可爱。
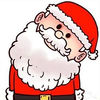
TA贡献1862条经验 获得超7个赞
我同意 Charles Duffy 的评论,即我们不为您设计程序。然而,你走错了路,我认为你需要一些指导。这是我正在谈论的一个例子。有很多方法可以做到这一点,这是一个简单的解决方案(众多解决方案之一)。
def pigLatin(word):
vowels = list("aeiou")
consonants = list("bcdfghjklmnpqrstvwxyz")
if word[0] in vowels:
word = word + "ay"
else:
for counter, letter in enumerate(list(word)):
if letter in vowels:
word = word[counter:] + word[:counter] + "ay"
break
return word
print(pigLatin("art"))
print(pigLatin("donkey"))
如果传递给 pigLatin 的单词包含大写字符怎么办?您可以通过将所有内容转换为小写(或大写,您的偏好)来修改该函数。
def pigLatin(word):
vowels = list("aeiou")
consonants = list("bcdfghjklmnpqrstvwxyz")
if word[0].lower() in vowels:
word = word + "ay"
else:
for counter, letter in enumerate(list(word)):
if letter.lower() in vowels:
word = word[counter:] + word[:counter] + "ay"
break
return word
您知道这段代码有多简单和灵活吗?
添加回答
举报