2 回答
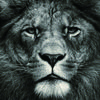
TA贡献1869条经验 获得超4个赞
可能存在一些更好的魔法解决方案,但我想最直接的解决方案是这样的
private static int[][] GetArrays(int[] intputArray)
{
// use lists for dynamically adding elements
var outputLists = new List<List<int>>();
// initialize with the ignored value
var lastValue = -1;
// iterate over the inputArray
foreach (var value in intputArray)
{
// skip -1 values
if (value < 0)
{
lastValue = -1;
continue;
}
// if a new value begin a new list
if (lastValue != value)
{
outputLists.Add(new List<int>());
}
// add the value to the current (= last) list
outputLists[outputLists.Count - 1].Add(value);
// update the lastValue
lastValue = value;
}
// convert to arrays
// you could as well directly return the List<List<int>> instead
// and access the values exactly the same way
// but since you speak of buffers I guess you wanted arrays explicitely
var outputArrays = new int[outputLists.Count][];
for (var i = 0; i < outputLists.Count; i++)
{
outputArrays[i] = outputLists[i].ToArray();
}
return outputArrays;
}
如此呼唤
var arrays = GetArrays(new int[]{1, 1, 1, 1, -1, -1, -1, -1, 0, 0, 0, 0, -1, -1, -1, 0, 0, 0});
应该导致
arrays[0] => [1, 1, 1, 1]
arrays[1] => [0, 0, 0, 0]
arrays[2] => [0, 0, 0]
因为您似乎更想动态地一一添加值,所以我根本不会使用数组,而是使用类似的东西
private List<List<int>> arrays = new List<List<int>>();
private int lastValue;
private void AddValue(int value)
{
// skip -1 values
if (value < 0)
{
lastValue = -1;
return;
}
// if a new value begin a new list
if (lastValue != value)
{
arrays.Add(new List<int>());
}
// add the value to the current (= last) list
arrays[outputLists.Count - 1].Add(value);
// update the lastValue
lastValue = value;
}
我的意思是你必须存储在某个地方的东西
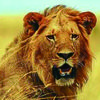
TA贡献1803条经验 获得超3个赞
不幸的是我没有找到任何开箱即用的解决方案,所以我想出了我自己的 Observable 实现:
using System;
using System.Collections.Generic;
using UniRx;
public static class ObservableFunctions
{
public static IObservable<T[]> BufferWhen<T>(this IObservable<T> source, Predicate<T> predicate)
{
return Observable.Create<T[]>(observer =>
{
List<T> buffer = new List<T>();
source.Subscribe(
t =>
{
if (predicate(t))
{
buffer.Add(t);
}
else
{
if (buffer.Count > 0)
{
observer.OnNext(buffer.ToArray());
buffer = new List<T>();
}
}
},
e =>
{
observer.OnError(e);
},
() =>
{
observer.OnCompleted();
}
);
return Disposable.Empty;
});
}
}
幸运的是,事情非常简单。比在 Google 中搜索适当的功能更简单...
- 2 回答
- 0 关注
- 101 浏览
添加回答
举报