1 回答
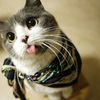
TA贡献1811条经验 获得超6个赞
这是一个方法,它将接受输入字符串,并通过查找“单词”(任何连续的非空格)来构建一个新字符串,然后检查该单词是否在字典中,如果找到,则将其替换为相应的值。Replace这将解决“子词”替换的问题(如果你有“hello hell”并且你想用“heaven”替换“hell”并且你不希望它给你“heaveno heaven”)。它还解决了交换问题。例如,如果您想将“yes no”中的“yes”替换为“no”,将“no”替换为“yes”,您不希望它首先将其变为“no no”,然后再变为“yes yes”。
public string ReplaceWords(string input, Dictionary<string, string> replacements)
{
var builder = new StringBuilder();
int wordStart = -1;
int wordLength = 0;
for(int i = 0; i < input.Length; i++)
{
// If the current character is white space check if we have a word to replace
if(char.IsWhiteSpace(input[i]))
{
// If wordStart is not -1 then we have hit the end of a word
if(wordStart >= 0)
{
// get the word and look it up in the dictionary
// if found use the replacement, if not keep the word.
var word = input.Substring(wordStart, wordLength);
if(replacements.TryGetValue(word, out var replace))
{
builder.Append(replace);
}
else
{
builder.Append(word);
}
}
// Make sure to reset the start and length
wordStart = -1;
wordLength = 0;
// append whatever whitespace was found.
builder.Append(input[i]);
}
// If this isn't whitespace we set wordStart if it isn't already set
// and just increment the length.
else
{
if(wordStart == -1) wordStart = i;
wordLength++;
}
}
// If wordStart is not -1 then we have a trailing word we need to check.
if(wordStart >= 0)
{
var word = input.Substring(wordStart, wordLength);
if(replacements.TryGetValue(word, out var replace))
{
builder.Append(replace);
}
else
{
builder.Append(word);
}
}
return builder.ToString();
}
- 1 回答
- 0 关注
- 254 浏览
添加回答
举报