1 回答
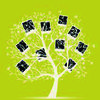
TA贡献1891条经验 获得超3个赞
那么您想从两个选择器获取状态并做一些工作,然后返回结果?这是 的完美问题类型reselect。Reselect 是一个帮助程序,可让您记住有关状态选择器的昂贵计算。
https://github.com/reduxjs/reselect
这对你来说可能是这样的。
$ yarn add reselect
import React from 'react';
import { useSelector } from 'react-redux';
import { createSelector } from 'reselect';
const filterArraySelector = (state) => state.filterArray;
const doctorsSelector = (state) => state.doctors;
const filteredDoctorsSelector = createSelector(doctorsSelector, filterArraySelector, (filterArray, doctors) => {
return doctors.filter((doctor) => {
return filterArray.all((key) => {
// Do some comparison here, return true if you want to include the doctor in the results
return doctor[key] !== undefined;
});
});
});
const Results = () => {
const filteredDoctors = useSelector(filteredDoctorsSelector);
return filteredDoctors.map((doctor) => <span>{doctor}</span>);
};
替代方案
您可以在每次渲染时简单地过滤医生,而不是使用createSelector记忆过滤。像这样:
const Results = () => {
const filterArray = useSelector((state) => state.filterArray);
const doctors = useSelector((state) => state.doctors);
const filteredDoctors = useMemo(
() =>
doctors.filter((doctor) => {
return filterArray.all((key) => {
// Do some comparison here, return true if you want to return the doctor
return doctor[key] !== undefined;
});
}),
[doctors, filterArray]
);
return filteredDoctors.map((doctor) => <span>{doctor}</span>);
};
给定一个像这样的值的filterArray:
const filterArray = ['Female', 'English'];
我们可以更新过滤器函数来根据 filterArray 值测试对象值。如果任何属性值与filterArray值匹配,那么我们可以将医生包含在生成的filteredDoctors列表中。
const Results = () => {
const filterArray = useSelector((state) => state.filterArray);
const doctors = useSelector((state) => state.doctors);
const filteredDoctors = useMemo(() => {
return doctors.filter((doctor) => {
return filterArray.some((filter) => {
return Object.values(doctor).some((value) => value === filter);
});
});
}, [doctors, filterArray]);
return filteredDoctors.map((doctor) => <span>{doctor}</span>);
};
更新:
const Results = () => {
const filterArray = useSelector((state) => state.filterArray);
const doctors = useSelector((state) => state.doctors);
const filteredDoctors = useMemo(() => {
return doctors.filter((doctor) => {
return filterArray.some((filter) => {
return Object.values(doctor).some((value) => {
// If the attribute value is an array
if (Array.isArray(value)) {
return value.some((value) => value === filter);
}
// If the attribute value is an object, get the values from the object
if (typeof value === 'object') {
return Object.values(value).some((value) => value === filter);
}
// By default, expect the value to be a string
return value === filter;
});
});
});
}, [doctors, filterArray]);
return filteredDoctors.map((doctor) => <span>{doctor}</span>);
};
添加回答
举报