4 回答
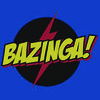
TA贡献1804条经验 获得超2个赞
映射缩减方法:
from itertools import groupby
from operator import itemgetter
scores = [['q1', 20],['q2',30],['q1',40],['q2',10],['q2',45],['q1',10]]
name, score = itemgetter(0), itemgetter(1)
grouped_scores = groupby(sorted(scores), key=name) # group by key
highest_scores = (max(g, key=score) for _,g in grouped_scores) # reduce by key
lowest_highest = min(highest_scores, key=score) # reduce
print(lowest_highest)
输出:
['q1', 40]
解释
和生成器表达式的返回值groupby
不是列表,如果您尝试直接打印它们,您会看到一堆无用的<itertools._grouper object at 0x7ff18bbbb850>
. 但是使用将每个不可打印对象转换为列表list()
,计算出的中间值如下:
scores = [['q1', 20],['q2',30],['q1',40],['q2',10],['q2',45],['q1',10]]
grouped_scores = [
['q1', [['q1', 10], ['q1', 20], ['q1', 40]]],
['q2', [['q2', 10], ['q2', 30], ['q2', 45]]]
]
highest_scores = [['q1', 40], ['q2', 45]]
lowest_highest = ['q1', 40]
Python 的map
和reduce
在本例中,我们正在寻找最高分数中的最低分数,因此在比较两个元素时,我们希望保留两者中的最小值。但在 python 中,我们可以直接调用整个序列,而不是min()
重复应用该函数。reduce
min()
仅供参考,如果我们使用的话,代码将如下所示reduce
:
from itertools import groupby
from functools import reduce
scores = [['q1', 20],['q2',30],['q1',40],['q2',10],['q2',45],['q1',10]]
name, score = itemgetter(0), itemgetter(1)
grouped_scores = groupby(sorted(scores), key=name) # group by key
highest_scores = map(lambda x: max(x[1], key=score), grouped_scores) # reduce by key
lowest_highest = reduce(lambda x,y: min(x,y, key=score), highest_scores) # reduce
print(lowest_highest)
输出:
['q1', 40]
使用模块 more_itertools
模块 more_itertools 有一个名为map_reduce的函数,它按键分组,然后按键减少。这照顾了我们的groupby
和max
步骤;我们只需要减少min
就可以得到我们的结果。
from more_itertools import map_reduce
from operator import itemgetter
scores = [['q1', 20],['q2',30],['q1',40],['q2',10],['q2',45],['q1',10]]
name, score = itemgetter(0), itemgetter(1)
highest_scores = map_reduce(scores, keyfunc=name, valuefunc=score, reducefunc=max)
lowest_highest = min(highest_scores.items(), key=score)
print(lowest_highest)
# ('q1', 40)
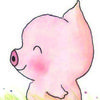
TA贡献1900条经验 获得超5个赞
好吧,如果您愿意pandas
,那么:
import pandas as pd
l = [["quizTitle1", 15],
["quizTitle2", 25],
["quizTitle1", 11],
["quizTitle3", 84],
["quizTitle2", 24]]
df = pd.DataFrame(l, columns=["quiz", "score"])
print(df)
# quiz score
# 0 quizTitle1 15
# 1 quizTitle2 25
# 2 quizTitle1 11
# 3 quizTitle3 84
# 4 quizTitle2 24
lowest_score = df.iloc[df.groupby(['quiz']).max().reset_index()["score"].idxmin()]
print(lowest_score)
# quiz quizTitle1
# score 15
# Name: 0, dtype: object
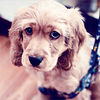
TA贡献1883条经验 获得超3个赞
一个简单的:
scores = [['q1', 20],['q2',30],['q1',40],['q2',10],['q2',45],['q1',10]]
d = dict(sorted(scores))
print(min(d, key=d.get)) # prints q1
该dict函数采用键/值对,我们只需要首先对它们进行排序,以便每个键的最后一个值是它最大的(因为最后一个是最终出现在字典中的值)。之后,所需的结果就是具有最小值的键。
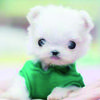
TA贡献1865条经验 获得超7个赞
您可以使用字典来存储每个测验的值,并用列表中迄今为止看到的最大值更新其值,然后获取字典中所有值的最小值。
scores = [['q1', 20],['q2',30],['q1',40],['q2',10],['q2',45],['q1',10]]
d = {}
for s in scores:
d[s[0]] = s[1] if s[0] not in d else max(d[s[0]], s[1])
print(d)
print("Lowest best : ", min(d.values()))
这打印:
{'q1': 40, 'q2': 45}
Lowest best : 40
添加回答
举报