3 回答
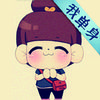
TA贡献1770条经验 获得超3个赞
from itertools import combinations
import numpy as np
N=9
# your set of numbers
nums = np.array([2,7,11,15])
# prepare list of all possible combinations of two numbers using built-in generator
combs = [i for i in combinations(nums,2)]
# sum up these two numbers
sums = np.sum(combs, axis=1)
# find index of wanted summed of the two numbers in sums
good_comb = np.where(sums==N)[0][0]
# search the indices in your original list, knowing index in combs
indices_sum_to_N = [np.where(nums==i)[0][0] for i in combs[good_comb]]
打印(indices_sum_to_N)
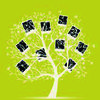
TA贡献1891条经验 获得超3个赞
您可以使用 enumerate 来获取符合您需要的条件的索引。下面的答案只是使用枚举的蛮力解决方案。它将返回列表列表。请注意,索引比较只是遍历对角线上的项目并且没有重复(例如,如果 [1,2] 在结果 [2,1] 中则不会)。
result = []
for index1, value1 in enumerate(items):
for index2, value2 in enumerate(items):
if index1>index2 and (value1 + value2)==target:
result.append([index1, index2])
print(result)
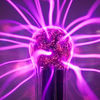
TA贡献1886条经验 获得超2个赞
像这种将值和索引作为字典返回的蛮力方法怎么样?
import itertools
def get_indexs_of_sum(search_list, search_val):
#remove any values higher than the search val
search_list = [val for val in search_list if val <= search_val]
#get all combinations with 2 elements
combinations = list(itertools.combinations(search_list, 2))
#check which lines sum the search val
totals = [comb for comb in combinations if sum(comb) == search_val]
#get indexes of answers
answerDict = {}
#loop answers get indexes and store in a dictionary
for i,answer in enumerate(totals):
indexes = []
for list_item in answer:
indexes.append(search_list.index(list_item))
answerDict[i] = {"Values":answer,"Index":indexes}
return answerDict
#GET THE LIST AND THE SEARCH VALUE
search_list = [19,15,1,13,1,3,16,7,11,10,16, 12, 18, 3, 15, 18, 7, 17, 6, 1, 2, 17, 1, 4, 12, 2, 14, 8, 10, 9, 19, 1, 18, 2, 3, 18, 3, 6, 6, 9]
search_val = 21
#call the function
answers = get_indexs_of_sum(search_list, search_val)
和输出
{0: {'Values': (19, 2), 'Index': [0, 20]},
1: {'Values': (19, 2), 'Index': [0, 20]},
2: {'Values': (19, 2), 'Index': [0, 20]},
3: {'Values': (15, 6), 'Index': [1, 18]},
4: {'Values': (15, 6), 'Index': [1, 18]},
5: {'Values': (15, 6), 'Index': [1, 18]},
6: {'Values': (13, 8), 'Index': [3, 27]},
7: {'Values': (3, 18), 'Index': [5, 12]},
8: {'Values': (3, 18), 'Index': [5, 12]},
9: {'Values': (3, 18), 'Index': [5, 12]},
10: {'Values': (3, 18), 'Index': [5, 12]},
11: {'Values': (7, 14), 'Index': [7, 26]},
12: {'Values': (11, 10), 'Index': [8, 9]},
13: {'Values': (11, 10), 'Index': [8, 9]},
14: {'Values': (12, 9), 'Index': [11, 29]},
15: {'Values': (12, 9), 'Index': [11, 29]},
16: {'Values': (18, 3), 'Index': [12, 5]},
17: {'Values': (18, 3), 'Index': [12, 5]},
18: {'Values': (18, 3), 'Index': [12, 5]},
19: {'Values': (3, 18), 'Index': [5, 12]},
20: {'Values': (3, 18), 'Index': [5, 12]},
21: {'Values': (3, 18), 'Index': [5, 12]},
22: {'Values': (15, 6), 'Index': [1, 18]},
23: {'Values': (15, 6), 'Index': [1, 18]},
24: {'Values': (15, 6), 'Index': [1, 18]},
25: {'Values': (18, 3), 'Index': [12, 5]},
26: {'Values': (18, 3), 'Index': [12, 5]},
27: {'Values': (7, 14), 'Index': [7, 26]},
28: {'Values': (17, 4), 'Index': [17, 23]},
29: {'Values': (2, 19), 'Index': [20, 0]},
30: {'Values': (17, 4), 'Index': [17, 23]},
31: {'Values': (12, 9), 'Index': [11, 29]},
32: {'Values': (12, 9), 'Index': [11, 29]},
33: {'Values': (2, 19), 'Index': [20, 0]},
34: {'Values': (19, 2), 'Index': [0, 20]},
35: {'Values': (18, 3), 'Index': [12, 5]},
36: {'Values': (18, 3), 'Index': [12, 5]},
37: {'Values': (3, 18), 'Index': [5, 12]},
38: {'Values': (18, 3), 'Index': [12, 5]}}
或者没有 itertools:
def get_indexs_of_sum_no_itertools(search_list, search_val):
answers = []
#loop list once
for i,x in enumerate(search_list):
#loop list twice
for ii,y in enumerate(search_list):
#if indexes aren't dupes and value matches:
if x != y and x + y == search_val:
answers.append({"indexes":(i,ii),"values":(x,y)})
return answers
search_list = [19,15,1,13,1,3,16,7,11,10,16, 12, 18, 3, 15, 18, 7, 17, 6, 1, 2, 17, 1, 4, 12, 2, 14, 8, 10, 9, 19, 1, 18, 2, 3, 18, 3, 6, 6, 9]
search_val = 14
ans = get_indexs_of_sum_no_itertools(search_list,search_val)
添加回答
举报