4 回答
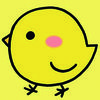
TA贡献1906条经验 获得超10个赞
下面的代码没有创建任何额外的数据结构。对于每个元素,它都会计算之前遇到的重复项的数量,并且只打印第一个重复项。
如果我在现实世界中这样做,我会使用 aSet但我假设您还没有了解它们,所以我只使用您已经创建的数组。
import java.util.Scanner;
public class DuplicateArray {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the size of the array: ");
int x = sc.nextInt();
int[] arr = new int[x];
System.out.print("Enter " + x + " values: ");
for (int i = 0; i < x; i++) {
arr[i] = sc.nextInt();
}
System.out.print("Array: ");
for (int i = 0; i < x; i++) {
System.out.print(arr[i]+" ");
}
System.out.println();
System.out.print("Duplicate elements:");
for (int i = 0; i < arr.length; i++) {
int numDups = 0;
for (int j = 0; j < i; j++) {
if (arr[i] == arr[j]) {
numDups++;
}
}
if (numDups == 1) {
System.out.print(" " + arr[i]);
}
}
System.out.println();
}
}
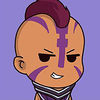
TA贡献1831条经验 获得超9个赞
如果不使用 Hashmap,我认为您最好的选择是首先对数组进行排序,然后计算重复项。由于数组现在有序,您可以在每次数字切换后打印重复项!
如果这是一项任务,请继续使用谷歌冒泡排序并将其实现为一种方法。
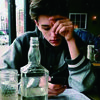
TA贡献1828条经验 获得超6个赞
System.out.println("Duplicate Elements : ");
for(int i = 0; i<arr.length; i++){
boolean isDuplicate = false;
for(int k=0;k<i;k++){
if(arr[i]== arr[k]){
isDuplicate = true;
break;
}
}
if(isDuplicate){
continue;
}
int count = 0;
for(int j=0; j<arr.length; j++){
if(arr[i] == arr[j]){
count++;
}
if(count >1){
System.out.println(arr[i]);
break;
}
}
}
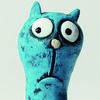
TA贡献1802条经验 获得超5个赞
一种解决方案是创建一个单独的列表来存储找到的任何重复项。
也就是说,除了使用 List 的 .contains() 方法之外,您还可以确保每个 int 只创建一个条目。
public static void main(String[] args) {
// Sample array of ints
int[] ints = {1, 1, 4, 5, 2, 34, 7, 5, 3};
// Create a separate List to hold duplicated values
List<Integer> duplicates = new ArrayList<>();
// Find duplicates
for (int i = 0; i < ints.length; i++) {
for (int j = 0; j < ints.length; j++) {
if (ints[i] == ints[j] && // Are the ints the same value?
i != j && // Ignore if we're looking at the same index
!duplicates.contains(ints[i])) { // Check if our List of duplicates already has this entry
duplicates.add(ints[i]); // Add to list of duplicates
}
}
}
System.out.println("Duplicates: " + duplicates);
}
输出:
Duplicates: [1, 5]
添加回答
举报