3 回答
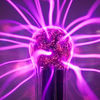
TA贡献1827条经验 获得超8个赞
如果您知道要替换的子字符串的长度,那么您可以迭代该字符串并检查该长度的所有可能子字符串,就像您通过“窗口”查看一样:
function replace(full, partial, placeholder) {
for (let i = 0; i <= full.length - partial.length; i++) {
const current = full.substring(i, i + partial.length);
if (current === partial) {
const prefix = full.substring(0, i);
const suffix = full.substring(i + partial.length);
return `${prefix}${placeholder}${suffix}`;
}
}
}
const ans = replace('abcdefghij', 'def', 'DENIED');
console.log(ans);
如果你想替换所有出现的地方,只是不要在第一次匹配后返回值:
function replaceAll(full, partial, placeholder) {
let tmp = full;
for (let i = 0; i <= tmp.length - partial.length; i++) {
const current = tmp.substring(i, i + partial.length);
if (current === partial) {
const prefix = tmp.substring(0, i);
const suffix = tmp.substring(i + partial.length);
tmp = `${prefix}${placeholder}${suffix}`;
i += placeholder.length;
}
}
return tmp;
}
const ans = replaceAll('abcdefghijdef', 'def', 'DENIED');
console.log(ans);
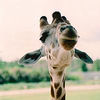
TA贡献1776条经验 获得超12个赞
由于您没有指定是要进行完全替换还是单数替换,因此我修改了它以允许使用一个boolean参数,这boolean说明是进行单数替换还是完全替换。
const replaceword = "DENIED";
const testword = "abcdef";
const testword2 = "abcdefdexyz";
const testword3 = "hello I don't have the sub";
//function takes a word parameter - the word to do a replace on
//and sub parameter of what to replace
//and replacement parameter of what to replace the substring with
//replaceall is a boolean as to whether to do a full replace or singular
function replace(word, sub, replacement, replaceall){
replaceall = replaceall || false; //default to singular replace
//Get the first index of the sub to replace
const startOfReplace = word.indexOf(sub);
//get the ending index of where the substring to be replaced ends
const endOfReplace = startOfReplace + sub.length - 1;
//variable to hold new word after replace
let replacedWord = "";
//If the substring is found do the replacement with the given replacement word
if(startOfReplace > -1){
for(let i = 0; i < word.length; i++){
if(i == startOfReplace){
replacedWord += replacement;
}else if(i >= startOfReplace && i <= endOfReplace){
continue;
}else{
replacedWord += word[i];
}
}
}else{ //set the return to the passed in word as no replacement can be done
replacedWord = word;
return replacedWord;
}
if(replaceall) //if boolean is true, recursively call function to replace all occurrences
//recursive call if the word has the sub more than once
return replace(replacedWord, sub, replacement);
else
return replacedWord; //else do the singular replacement
}
console.log(replace(testword, "de", replaceword));
console.log(replace(testword2, "de", replaceword, true));
console.log(replace(testword3, "de", replaceword));
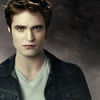
TA贡献1848条经验 获得超6个赞
const source = "abcdefg";
const target = "bcd";
const replacer = "DENIED";
const replace = (source, target, replacer) => {
const position = source.indexOf(target);
if(position === -1) return source;
let output = source.substr(0, position)
output += replacer
output += source.substr(position + target.length);
return output
}
const replaceAll = (source, target, replacer) => {
let output = source;
do{
output = replace(output, target, replacer)
}
while(output !== replace(output, target, replacer))
return output;
}
console.log(replace(source, target, replacer))
并且可以肯定的是,最好的解决方案,最容易理解,干净和优雅的是:
const replaceAll = (source, target, replacer) => {
return source.split(target).join(replacer)
}
添加回答
举报