4 回答
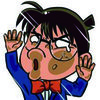
TA贡献1872条经验 获得超3个赞
此方法使用 javascript 识别哪个项目具有mouseover,然后将active类添加到之前的所有项目。这些元素将保留它们的active类,直到将鼠标悬停在会导致活动类被删除的元素上为止。
请注意,我还向您的<li>元素添加了数据属性,以便更轻松地处理它们:
let liArray = document.querySelectorAll("li");
liArray.forEach( (element) =>
element.addEventListener("mouseover",
function( event ) {
doHighlights(+event.target.dataset.step);
}
));
function doHighlights(inStep) {
let liArray = document.querySelectorAll("li");
//console.log(inStep);
liArray.forEach( (element) => {
//console.log(+element.dataset.step, inStep);
if (+element.dataset.step <= inStep) {
element.classList.add("active");
} else {
element.classList.remove("active");
}
})
}
@import "compass/css3";
li {
width: 2em;
height: 2em;
text-align: center;
line-height: 2em;
border-radius: 1em;
background: lightblue;
margin: 0 1em;
display: inline-block;
color: white;
position: relative;
}
li::before {
content: '';
position: absolute;
top: 0.9em;
left: -4em;
width: 4em;
height: 0.2em;
background: lightblue;
z-index: -1;
}
li.active::before {
background: dodgerblue;
}
li:first-child::before {
display: none;
}
li.active {
background: dodgerblue;
}
body {
font-family: sans-serif;
padding: 2em;
}
/*
.active ~ li {
background: lightblue;
}
.active ~ li::before {
background: lightblue;
}
*/
/*
li:hover {
background: dodgerblue;
}
*/
<ul>
<li data-step="1">1</li>
<li data-step="2">2</li>
<li data-step="3">3</li>
<li data-step="4">4</li>
<li data-step="5">5</li>
<li data-step="6">6</li>
<li data-step="7">7</li>
</ul>
(此处为 JSFiddle 版本:https://jsfiddle.net/79hwa6s4/)
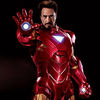
TA贡献2019条经验 获得超9个赞
这很容易!您所要做的就是从HTML 文件的 li 标签中删除active (Class) ,并在 CSS 文件中将.active替换为li:hover。
JSFiddle :: https://jsfiddle.net/chbej806/2/
变化
=> HTML
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
</ul>
=> CSS
@import "compass/css3";
li {
width: 2em;
height: 2em;
text-align: center;
line-height: 2em;
border-radius: 1em;
background: dodgerblue;
margin: 0 1em;
display: inline-block;
color: white;
position: relative;
}
li::before {
content: '';
position: absolute;
top: 0.9em;
left: -4em;
width: 4em;
height: 0.2em;
background: dodgerblue;
z-index: -1;
}
li:first-child::before {
display: none;
}
li:hover{
background: dodgerblue;
cursor: pointer;
}
li:hover ~ li {
background: lightblue;
}
li:hover ~ li::before {
background: lightblue;
}
body {
font-family: sans-serif;
padding: 2em;
}
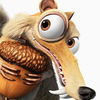
TA贡献1810条经验 获得超4个赞
activeIndex = -1;
$("#my-progress li").each(function(index){
if($(this).hasClass("active"))
activeIndex = index;
})
$("#my-progress li").mouseover(function(index){
$("#my-progress li").removeClass("active");
$(this).addClass("active");
})
$("#my-progress li").mouseout(function(index){
$("#my-progress li").removeClass("active");
$($("#my-progress li")[activeIndex]).addClass("active");
})
li {
width: 2em;
height: 2em;
text-align: center;
line-height: 2em;
border-radius: 1em;
background: dodgerblue;
margin: 0 1em;
display: inline-block;
color: white;
position: relative;
cursor:pointer
}
li::before {
content: '';
position: absolute;
top: 0.9em;
left: -4em;
width: 4em;
height: 0.2em;
background: dodgerblue;
z-index: -1;
}
li:first-child::before {
display: none;
}
.active {
background: dodgerblue;
}
.active ~ li {
background: lightblue;
}
.active ~ li::before {
background: lightblue;
}
body {
font-family: sans-serif;
padding: 2em;
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<ul id="my-progress">
<li>1</li>
<li>2</li>
<li>3</li>
<li class="active">4</li>
<li>5</li>
<li>6</li>
<li>7</li>
</ul>
如果你想将鼠标悬停在项目上冻结而不是恢复到最后状态......从你的脚本中删除以下代码:
$("#my-progress li").mouseout(function(index){
$("#my-progress li").removeClass("active");
$($("#my-progress li")[activeIndex]).addClass("active");
})
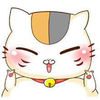
TA贡献1864条经验 获得超2个赞
好吧,你为什么不为此使用 JavaScript,只是为了改变哪个<li>元素有类"active",这里是你如何做到的,我写了一些评论来帮助你理解代码
// an event delegation for the ul on hover
document.querySelector("ul").onmouseover = function(e) {
// get the current active <li> element to disactivate it later
let element = this.querySelector(".active");
// if the current hovered element is a <li> element
if(e.target.nodeName === "LI") {
// just activate the current hovered element
e.target.classList.add("active");
// and disactivate the old one only if we found one
element !== e.target && (element.classList.remove("active"));
}
}
@import "compass/css3";
li {
width: 2em;
height: 2em;
text-align: center;
line-height: 2em;
border-radius: 1em;
background: dodgerblue;
margin: 0 1em;
display: inline-block;
color: white;
position: relative;
}
li::before {
content: '';
position: absolute;
top: 0.9em;
left: -4em;
width: 4em;
height: 0.2em;
background: dodgerblue;
z-index: -1;
}
li:first-child::before {
display: none;
}
.active {
background: dodgerblue;
}
.active ~ li {
background: lightblue;
}
.active ~ li::before {
background: lightblue;
}
body {
font-family: sans-serif;
padding: 2em;
}
<ul>
<li class="active">1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
</ul>
添加回答
举报