1 回答
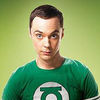
TA贡献1744条经验 获得超4个赞
我假设这是您在选择您是用户还是司机后到达的部分。如果我们坚持我在你的另一篇文章中给你的例子:
新配置.py
from dataclasses import dataclass, asdict
#to make things more complicated and force you to get better
#create a dataclass of default properties
@dataclass
class Button_dc:
bg: str = "white"
fg: str = "gray20"
font: str = 'Calibri 14 bold'
border:int = 10
#make a dict instance of the dataclass
DefaultButton = asdict(Button_dc())
#make a slightly different instance
UserRoleButton = asdict(Button_dc(font='Calibri 24 bold'))
@dataclass
class Label_dc:
bg: str = "steel blue"
fg: str = "gray20"
font: str = 'Calibri 14 bold'
DefaultLabel = asdict(Label_dc())
@dataclass
class Entry_dc:
bg: str = "white"
fg: str = "gray20"
font: str = 'Calibri 14 bold'
width: int = 30
DefaultEntry = asdict(Entry_dc())
@dataclass
class Message_dc:
bg: str = "red"
fg: str = "white"
font: str = 'Calibri 14 bold'
width: int = 150
DefaultMessage = asdict(Message_dc())
在main.py中选择整个user_form方法并将下面的整个粘贴到它的位置
def user_form(self, type, fname=None, lname=None):
self.canvas.delete("all")
self.canvas.create_window(10, 10, anchor='nw', window=tk.Label(self, text="First Name:", **cfg.DefaultLabel))
fname_ent = tk.Entry(self, **cfg.DefaultEntry)
if fname != None:
fname_ent.insert('end', fname)
self.canvas.create_window(120, 10, anchor='nw', window=fname_ent)
self.canvas.create_window(10, 40, anchor='nw', window=tk.Label(self, text="Last Name:", **cfg.DefaultLabel))
lname_ent = tk.Entry(self, **cfg.DefaultEntry)
if lname != None:
lname_ent.insert('end', lname)
self.canvas.create_window(120, 40, anchor='nw', window=lname_ent)
#don't send the entire Entry to the check ~ only the data you want to check
form_next_btn = tk.Button(self, text="next", command=lambda: self.check(type, fname_ent.get(), lname_ent.get()), **cfg.DefaultButton)
self.fnb = self.canvas.create_window(500, 340, anchor='nw', window=form_next_btn)
def check(self, type, fname, lname):
self.canvas.delete(self.fnb)
f = fname.isalpha()
l = lname.isalpha()
if f and l:
self.process(type, fname, lname)
return
if not f:
errormsg = tk.Message(self, text='Invalid entry for first name', **cfg.DefaultMessage)
self.canvas.create_window(10, 90, anchor='nw', window=errormsg)
if not l:
errormsg2 = tk.Message(self, text='Invalid entry for last name', **cfg.DefaultMessage)
self.canvas.create_window(10 if f else 170, 90, anchor='nw', window=errormsg2)
back_btn = tk.Button(self, text="back", command=lambda: self.user_form(type, fname if f else None, lname if l else None), **cfg.DefaultButton)
self.canvas.create_window(500, 340, anchor='nw', window=back_btn)
def process(self, type, fname, lname):
self.canvas.delete("all")
if type is Role.User:
print(f'{fname} {lname} is a user')
elif type is Role.Driver:
print(f'{fname} {lname} is a driver')
旁白:请记住,我根本没有尝试让您的应用程序漂亮。我只是向您展示如何让它发挥作用。我也没有写出我最好的代码。我会做所有这些完全不同的事情。我正在讨论你的canvas方法并给你一些理智的东西。在我看来,您使用画布的全部原因只是为了拥有一个圆角矩形。这似乎不值得,但这不是我的决定。
然而,这些概念并不糟糕,而且在适当的上下文中可以非常强大。让我们以dataclasses, 为例。想象一下,如果您真的想执行以下操作。您最终会得到一个完全自定义的Text小部件和一种非常简单的方法来仅更改您需要更改的内容,同时保留您分配的所有其他内容。我dataclasses为您编写的是一些示例概念。你可以把这些概念带得更远。
@dataclass
class Text_dc:
background: str = Theme.Topcoat # main element
foreground: str = Theme.Flat
borderwidth: int = 0
selectbackground: str = Theme.Primer # selected text
selectforeground: str = Theme.Accent
selectborderwidth: int = 0 # doesn't seem to do anything ~ supposed to be a border on selected text
insertbackground: str = Theme.Hilight # caret
insertborderwidth: int = 0 # border
insertofftime: int = 300 # blink off millis
insertontime: int = 600 # blink on millis
insertwidth: int = 2 # width
highlightbackground:int = Theme.Topcoat # inner border that is activated when the widget gets focus
highlightcolor: int = Theme.Topcoat # color
highlightthickness: int = 0 # thickness
cursor: str = 'xterm'
exportselection: int = 1
font: str = 'calibri 14'
width: int = 16 # characters ~ often this is ignored as Text gets stretched to fill its parent
height: int = 8 # lines ~ " " " " "
padx: int = 2
pady: int = 0
relief: str = 'flat'
wrap: str = 'word' # "none", 'word'
spacing1: int = 0 # space above every line
spacing2: int = 0 # space between every wrapped line
spacing3: int = 0 # space after return from wrap (paragraph)
state: str = 'normal' # NORMAL=Read/Write | DISABLED=ReadOnly
tabs: str = '4c'
takefocus: int = 1
undo: bool = True
xscrollcommand: Callable = None
yscrollcommand: Callable = None
#common difference
NoWrap = asdict(Text_dc(**{'wrap':'none'}))
NWReadOnly = asdict(Text_dc(**{'wrap':'none','state':'disabled'}))
ReadOnly = asdict(Text_dc(**{'state':'disabled'}))
DefaultText = asdict(Text_dc())
您可以以不同的方式使用该概念。假设您想要一个完全自定义的Text小部件,并且您打算做的不仅仅是重新设计它。下面是一个快速而肮脏的例子
class ScriptEditor(tk.Text):
def __init__(self, master, **kwargs):
tk.Text.__init__(self, master, **asdict(Text_dc(font='Consolas 14', **kwargs)))
def syntax_highlight(self):
pass
#all the other **kwargs are defaulted internally,
#but we want to adjust the size for this instance
editor = ScriptEditor(root, width=120, height=80)
添加回答
举报