5 回答
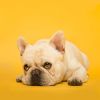
TA贡献1797条经验 获得超4个赞
您可以使用itertools.groupby
:
>>> from itertools import groupby
>>> result = []
>>> for key, group in groupby(sorted(sample, key=lambda x:x['a']), key=lambda x:x.pop('a')):
result.append({'a':key, 'd':[*group]})
>>> result
[{'a': 1, 'd': [{'b': 2, 'c': 3}, {'b': 2, 'c': 4}]},
{'a': 2, 'd': [{'b': 2, 'c': 5}, {'b': 3, 'c': 5}]}]
sorted注意:如果保证字典列表按 key 的值排序,则不需要a。
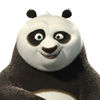
TA贡献1786条经验 获得超13个赞
按键组合:
dict_list = [{'a':1, 'b':2, 'c':3}, {'a':1, 'b':2, 'c':4}, {'a':2, 'b':2, 'c':5}, {'a':2, 'b':3, 'c':5}]
new_dict = {}
for d in dict_list:
a = d.pop('a', None)
if new_dict.get(a):
new_dict[a].append(d)
else:
new_dict[a] = [d]
转换为列表:
final_list = [{'a': key, 'd': value} for key, value in new_dict.items()]
print(final_list)
[{'a': 1, 'd': [{'c': 3, 'b': 2}, {'c': 4, 'b': 2}]}, {'a': 2, 'd': [{'c': 5, 'b': 2}, {'c': 5, 'b': 3}]}]
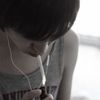
TA贡献1797条经验 获得超6个赞
sample = [{'a':1, 'b':2, 'c':3}, {'a':1, 'b':2, 'c':4}, {'a':2, 'b':2, 'c':5}, {'a':2, 'b':3, 'c':5}]
tmp = {}
for v in sample:
tmp.setdefault(v['a'], []).append(v)
del v['a']
out = [{'a': k, 'd': v} for k, v in tmp.items()]
from pprint import pprint
pprint(out)
印刷:
[{'a': 1, 'd': [{'b': 2, 'c': 3}, {'b': 2, 'c': 4}]},
{'a': 2, 'd': [{'b': 2, 'c': 5}, {'b': 3, 'c': 5}]}]
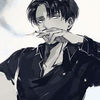
TA贡献1828条经验 获得超3个赞
不幸的是,这可能是一个有点曲折的代码,但它有效:
from itertools import groupby
sample = [{'a':1, 'b':2, 'c':3},
{'a':1, 'b':2, 'c':4},
{'a':2, 'b':2, 'c':5},
{'a':2, 'b':3, 'c':5}]
main_key = "a"
print(
[{main_key:k,
"d": [{kk: vv for kk, vv in dct.items() if kk != main_key}
for dct in v]}
for k, v in groupby(sample, lambda d:d[main_key])]
)
给出:
[{'a': 1, 'd': [{'b': 2, 'c': 3}, {'b': 2, 'c': 4}]},
{'a': 2, 'd': [{'b': 2, 'c': 5}, {'b': 3, 'c': 5}]}]
(为了便于阅读,输出稍微漂亮一点)
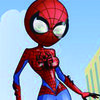
TA贡献1851条经验 获得超4个赞
使用 Pandas 进行查询的替代解决方案。
import pandas as pd
sample = [{'a':1, 'b':2, 'c':3}, {'a':1, 'b':2, 'c':4}, {'a':2, 'b':2, 'c':5}, {'a':2, 'b':3, 'c':5}]
df=pd.DataFrame(sample)
这将使用上面的示例列表创建一个 DataFrame df。下一步是遍历 GroupBy 对象并根据需要创建输出。
final_list=[]
for i, temp_df in df.groupby('a'):
temp_list=[]
for j in temp_df.index:
temp_list.append({'b':temp_df.loc[:,'b'][j],'c':temp_df.loc[:,'c'][j]})
final_list.append({'a':temp_df.loc[:,'a'][j],'d':temp_list})
添加回答
举报