4 回答
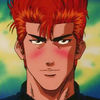
TA贡献1839条经验 获得超15个赞
您需要搜索的是 Javascript 中的 DOM 操作。这是一个简单的方法来做你所要求的。我试图尽可能地解释它。
<html>
<body>
<div id="animals">
<img src="cat.jpg" id="cat">
<img src="dog.jpg" id="dog">
<img src="frog.jpg" id="frog">
</div>
</body>
<script>
// animals variable here is a NodeList which is kind of like Array,
// but it is not enumerable (you cannot loop over it).
var animals = document.querySelectorAll('#animals > *');
// Here we convert it to an actual Array.
var animalsArray = Array.from(animals);
// We are going to add click listeners on every single of them.
animalsArray.forEach(function (animal) {
// Add an event listener to this img element.
animal.addEventListener(
// The listener should observe click events.
'click',
// The listener will call this function
// when a click event is triggered.
function (event) {
// This is the order number of the clicked animal image.
// Assuming that you have 3 images on the page, this number
// will be 0, 1 or 2.
// 0 marking the image as the first of rest, and 2 marking it
// as the last of them.
var positionOfThisAnimal = animalsArray.indexOf(this);
// animalsArray.length will give you the number of images in
// the `animalsArray` Array.
// If the position of the clicked animal image is smaller than
// the highest it can be (which is `2` for 3 images), it is not
// the last one, and we will alert the user with a message.
if (positionOfThisAnimal < animalsArray.length - 1) {
alert("Don't click me! Click the frog.");
// Because all we have to do for these not-the-last-one
// images is to alert the user, we are returning a void
// (empty) result here which effectively stops the function
// from executing the rest of the lines we have below.
return;
}
// Here we are taking the clicked animal image (which is the
// last one in the list) and we are moving it to the first
// place in the div with ID "animals".
document.querySelector('#animals').prepend(this);
},
// This `false` means the listener should not be marked as passive.
//
// Passive listeners are asynchronous, meaning that the browser
// will *not* wait for your function to complete executing before
// continuing to wait for other user inputs like mouse moves or
// keyboard strokes.
//
// The browser will wait for active listeners. This has a downside
// though. If your function takes too long to complete, the user
// will notice that their browser (or just the current tab) freezes
// and won't let user interact with the page until the execution
// is completed.
false,
);
});
</script>
</html>
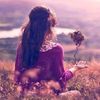
TA贡献1842条经验 获得超21个赞
您可以在用户单击图像时更改图像的来源,例如:
let cat = document.getElementById("cat");
let frog = document.getElementById("frog");
function moveFunction() {
if(frog.src == "frog.jpg"){
frog.src = "cat.jpg";
}
else {
frog.src = "frog.jpg";
}
}
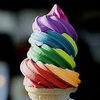
TA贡献1780条经验 获得超1个赞
const img1 = document.querySelector('#cat');
const img2 = document.querySelector('#dog');
const img3 = document.querySelector('#frog');
const imgUrl = img3.src;
img1.addEventListener('click', () => {
if(img1.src === imgUrl) {
let temp = img1.src;
img1.src = img2.src;
img2.src = img3.src;
img3.src = temp;
let temp2 = img1.id;
img1.id = img2.id;
img2.id = img3.id;
img3.id = temp2;
}
else {
alert('Don\'t click me, click the frog');
};
});
img2.addEventListener('click', () => {
alert('Don\'t click me, click the frog');
});
img3.addEventListener('click', () => {
if(img3.src === imgUrl) {
let temp = img1.src;
img1.src = img3.src;
img3.src = img2.src;
img2.src = temp;
let temp2 = img1.id;
img1.id = img3.id;
img3.id = img2.id;
img2.id = temp2;
}
else {
alert('Don\'t click me, click the frog');
};
});
img {
width: 300px;
height: 300px;
}
<div>
<img src="cat.jpg" id="cat">
<img src="dog.jpg" id="dog">
<img src="frog.jpg" id="frog">
</div>
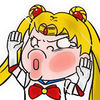
TA贡献1856条经验 获得超11个赞
我们可以在 CSS 中使用 flex 和 order 来做到这一点,检查下面的代码,而不是图像,我使用了带有颜色的 div。
.maindiv {
display: flex;
}
#frog {
padding: 10px;
width: 20px;
background: red;
}
#cat {
padding: 10px;
width: 20px;
background: blue;
}
#dog {
padding: 20px;
width: 10px;
background: green;
}
<html>
<body>
<div class="maindiv">
<div id="cat" onclick="alertfunction()">cat</div>
<div id="dog" onclick="alertfunction()">dog</div>
<div id="frog" onclick="movefunction()">frog</div>
</div>
</body>
<script>
function alertfunction() {
alert("Don't click me, click the frog");
}
function movefunction(){
if (document.getElementById('frog').style.order == '1') {
document.getElementById('frog').style.order = '3';
document.getElementById('cat').style.order = '1';
document.getElementById('dog').style.order = '2';
} else {
document.getElementById('frog').style.order = '1';
document.getElementById('cat').style.order = '2';
document.getElementById('dog').style.order = '3';
}
}
</script>
</html>
添加回答
举报