2 回答
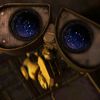
TA贡献2021条经验 获得超8个赞
您最好的选择可能是在 python 端创建数据,以便对其进行重新计数和垃圾收集。
test.py
import example
import numpy as np
array = np.zeros((3, 2, 4, 4), 'f4')
example.do_math(array, 3, 2)
print(array[0, 0])
例子.cpp
#define PY_SSIZE_T_CLEAN
#include <Python.h>
#include <Eigen/Dense>
Eigen::Matrix4f get_dummy() {
Eigen::Matrix4f mat_a;
mat_a << 1, 2, 3, 4,
5, 6, 7, 8,
9, 8, 7, 6,
5, 4, 3, 2;
return mat_a;
}
PyObject * example_meth_do_math(PyObject * self, PyObject * args, PyObject * kwargs) {
static char * keywords[] = {"array", "rows", "cols", NULL};
PyObject * array;
int rows, cols;
if (!PyArg_ParseTupleAndKeywords(args, kwargs, "Oii", keywords, &array, &rows, &cols)) {
return NULL;
}
Py_buffer view = {};
if (PyObject_GetBuffer(array, &view, PyBUF_SIMPLE)) {
return NULL;
}
Eigen::Matrix4f * ptr = (Eigen::Matrix4f *)view.buf;
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
ptr[i * cols + j] = get_dummy();
}
}
PyBuffer_Release(&view);
Py_RETURN_NONE;
}
PyMethodDef module_methods[] = {
{"do_math", (PyCFunction)example_meth_do_math, METH_VARARGS | METH_KEYWORDS, NULL},
{},
};
PyModuleDef module_def = {PyModuleDef_HEAD_INIT, "example", NULL, -1, module_methods};
extern "C" PyObject * PyInit_example() {
PyObject * module = PyModule_Create(&module_def);
return module;
}
setup.py
from setuptools import Extension, setup
ext = Extension(
name='example',
sources=['./example.cpp'],
extra_compile_args=['-fpermissive'],
include_dirs=['.'], # add the path of Eigen
library_dirs=[],
libraries=[],
)
setup(
name='example',
version='0.1.0',
ext_modules=[ext],
)
从这里添加第二个参数并使用两个数组进行计算应该是微不足道的。
您可以使用python setup.py develop.
如果你想分发它,你可以创建一个 wheel 文件python setup.py bdist_wheel。
我曾经numpy创建数据,这确保了数据的底层内存是 C 连续的。
这个例子很简单,它使用一个 Matrix4f 指针来迭代一个 3x2 矩阵数组。随意将 转换ptr为Eigen::Array<Eigen::Matrix4f>, 3, 2>。您不能将其强制转换为 an std::vector,因为 an 的内部数据std::vector包含指针。
请注意,std::vector<std::array<...>>内存中没有单个连续数组。改用Eigen::Array。
编辑:
这是一个使用Eigen Array Map:
PyObject * example_meth_do_math(PyObject * self, PyObject * args, PyObject * kwargs) {
static char * keywords[] = {"array", NULL};
PyObject * array;
if (!PyArg_ParseTupleAndKeywords(args, kwargs, "O", keywords, &array)) {
return NULL;
}
Py_buffer view = {};
if (PyObject_GetBuffer(array, &view, PyBUF_SIMPLE)) {
return NULL;
}
Eigen::Map<Eigen::Array<Eigen::Matrix4f, 2, 3>> array_map((Eigen::Matrix4f *)view.buf, 2, 3);
for (int i = 0; i < 2; ++i) {
for (int j = 0; j < 3; ++j) {
array_map(i, j) = get_dummy();
}
}
PyBuffer_Release(&view);
Py_RETURN_NONE;
}
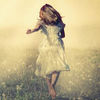
TA贡献1789条经验 获得超10个赞
线性代数不会那么流畅(在那里很难击败 Eigen),但会类似于您在 numpy 中所做的(np.dot(A,B)
例如。
如果您想坚持使用 Eigen,请注意使用 STL 有一些技术细节。由于您std::array
不再能够包含固定数量的矩阵,因此当您移动到std::vector
您会遇到对齐问题(诚然,我不完全理解)。很快就会为您提供 xtensor 的有效实现。
添加回答
举报