2 回答
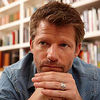
TA贡献1784条经验 获得超7个赞
在这里简化逻辑的一种方法可能是运行一个修改地图的 goroutine。然后,它可以监听通道上的新值(因为如果按顺序处理值应该没问题)。您需要小心知道您的 goroutine 何时返回以确保它不会泄漏。一般来说,你不应该在 goroutines 之间共享数据,你应该使用通道在 goroutines 之间进行通信。
这是一个示例,说明如何使用通道而不是共享内存(游乐场版本)来实现此类应用程序。
package main
import (
"fmt"
"sync"
)
type value struct {
key string
value float64
threshold float64
}
func main() {
b := board{
last: map[string]float64{},
}
c := b.start()
wg := sync.WaitGroup{}
for i := 0; i < 30; i++ {
wg.Add(1)
go func(i int) {
for j := 15.0; j > 5; j-- {
c <- value{"k1", j + float64(i+1)/100, 10}
}
wg.Done()
}(i)
}
wg.Wait()
close(c)
}
type board struct {
last map[string]float64
}
func (b *board) start() chan<- value {
c := make(chan value)
go func() {
for v := range c {
b.notify(v)
}
}()
return c
}
func (b *board) notify(v value) {
if l, ok := b.last[v.key]; !ok || l >= v.threshold {
if v.value < v.threshold {
fmt.Printf("%s < %v (%v)\n", v.key, v.threshold, v.value)
}
}
b.last[v.key] = v.value
}
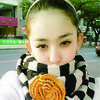
TA贡献1890条经验 获得超9个赞
我认为您在设置此类跟踪器时需要设置标志,一个用于价值上升,另一个用于价值下降。我实施了一个
package main
import (
"fmt"
"sync"
)
const (
threshold int = 10
upperThreshold int = 20
)
var mu sync.Mutex
var downwatch bool
var upwatch bool
func main() {
var tracker int = 10
var temp int = 1
var sign int = 1
for i := 1; i < 20; i++ {
sign = sign * -1
temp = temp + i
go UpdateTracker(&tracker, temp*sign)
}
for {
}
return
}
func SetDownWatch() {
downwatch = true
}
func SetUpWatch() {
upwatch = true
}
func UnSetDownWatch() {
downwatch = false
}
func UnSetUpWatch() {
upwatch = false
}
func UpdateTracker(tracker *int, val int) {
mu.Lock()
defer mu.Unlock()
if !(upwatch || downwatch) {
if (*tracker)+val < threshold {
NotifyOnDrop()
SetDownWatch()
}
if (*tracker + val) > upperThreshold {
NotifyOnRise()
SetUpWatch()
}
}
if (*tracker)+val < threshold && upwatch {
NotifyOnDrop()
SetDownWatch()
UnSetUpWatch()
}
if (*tracker+val) > upperThreshold && downwatch {
NotifyOnRise()
SetUpWatch()
UnSetDownWatch()
}
*tracker = (*tracker) + val
fmt.Println((*tracker))
return
}
func NotifyOnDrop() {
fmt.Println("dropped")
return
}
func NotifyOnRise() {
fmt.Println("rose")
return
}
updateTracker当值超过设置的阈值时,作为 go 例程运行并打印到控制台。我认为这就是您正在寻找的功能,这里缺少的是Last.Store我认为是您的代码自定义的功能。我相信还有其他方法可以处理这个问题。这对我来说似乎很简单。
- 2 回答
- 0 关注
- 154 浏览
添加回答
举报