1 回答
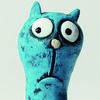
TA贡献1799条经验 获得超9个赞
享受这个通用的解决方案:
转换器类
Junit 测试——显示了 1 个没有名称空间的测试,但它确实适用于名称空间
使用 XSLT 剥离名称空间的转换器类。JAXB 有时过于直白,您可以在许多帖子中看到这一点。
public class XmlToPojo {
public static Object convertXmlToObject(String xmlString, Class targetClass) {
JAXBContext jaxbContext;
try {
// Step 1 - remove namespaces
StreamSource xmlSource = new StreamSource(new StringReader(xmlString));
StreamResult result = new StreamResult(new StringWriter());
removeNamespace(xmlSource, result);
// Step 2 - convert XML to object
jaxbContext = JAXBContext.newInstance(targetClass);
Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
return jaxbUnmarshaller.unmarshal(new StringReader(result.getWriter().toString()));
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
// Remove namespaces from XML
private static String xsltNameSpaceRemover = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n" +
"<xsl:stylesheet version=\"1.0\" xmlns:xsl=\"http://www.w3.org/1999/XSL/Transform\">\n" +
"\n" +
" <xsl:output indent=\"yes\" method=\"xml\" encoding=\"utf-8\" omit-xml-declaration=\"yes\"/>\n" +
"\n" +
" <!-- Stylesheet to remove all namespaces from a document -->\n" +
" <!-- NOTE: this will lead to attribute name clash, if an element contains\n" +
" two attributes with same local name but different namespace prefix -->\n" +
" <!-- Nodes that cannot have a namespace are copied as such -->\n" +
"\n" +
" <!-- template to copy elements -->\n" +
" <xsl:template match=\"*\">\n" +
" <xsl:element name=\"{local-name()}\">\n" +
" <xsl:apply-templates select=\"@* | node()\"/>\n" +
" </xsl:element>\n" +
" </xsl:template>\n" +
"\n" +
" <!-- template to copy attributes -->\n" +
" <xsl:template match=\"@*\">\n" +
" <xsl:attribute name=\"{local-name()}\">\n" +
" <xsl:value-of select=\".\"/>\n" +
" </xsl:attribute>\n" +
" </xsl:template>\n" +
"\n" +
" <!-- template to copy the rest of the nodes -->\n" +
" <xsl:template match=\"comment() | text() | processing-instruction()\">\n" +
" <xsl:copy/>\n" +
" </xsl:template>\n" +
"\n" +
"</xsl:stylesheet>";
private static void removeNamespace(Source xmlSource, Result xmlOutput) throws TransformerException {
TransformerFactory factory = TransformerFactory.newInstance();
StreamSource xsltSource = new StreamSource(new StringReader(xsltNameSpaceRemover));
Transformer transformer = factory.newTransformer(xsltSource);
transformer.transform(xmlSource, xmlOutput);
}
}
一个简单的 Junit 测试:
public class XmlToPojoTest {
@Test
public void testBasic() {
String xmlString = "<employee>" +
" <department>" +
" <id>101</id>" +
" <name>IT-ABC</name>" +
" </department>" +
" <firstName>JJ</firstName>" +
" <id>1</id>" +
" <lastName>JoHo</lastName>" +
"</employee>";
XmlToPojo xmlToPojo = new XmlToPojo();
Employee emp = (Employee) xmlToPojo.convertXmlToObject(xmlString, Employee.class);
assertEquals("JJ", emp.getFirstName());
assertEquals("IT-ABC", emp.getDepartment().getName());
}
}
添加回答
举报