3 回答
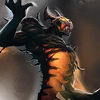
TA贡献1836条经验 获得超4个赞
如果要使用 JAVA Stream API,对象必须是 Iterable。正如@Michal 提到的,您可以将其更改为 Collection。从@Michal 的回答中,我们可以简化它,就像:
public class JsonArrayIntersectTest {
private boolean anyMatch(JSONArray first, JSONArray second) throws JSONException {
Set<Object> firstAsSet = convertJSONArrayToSet(first);
Set<Object> secondIdsAsSet = convertJSONArrayToSet(second);
// use stream API anyMatch
return firstAsSet.stream()
.anyMatch(secondIdsAsSet::contains);
}
private Set<Object> convertJSONArrayToSet(JSONArray input) throws JSONException {
Set<Object> retVal = new HashSet<>();
for (int i = 0; i < input.length(); i++) {
retVal.add(input.get(i));
}
return retVal;
}
@Test
public void testCompareArrays() throws JSONException {
JSONArray deviation = new JSONArray(ImmutableSet.of("1", "2", "3"));
JSONArray deviationIds = new JSONArray(ImmutableSet.of("3", "4", "5"));
Assert.assertTrue(anyMatch(deviation, deviationIds));
deviation = new JSONArray(ImmutableSet.of("1", "2", "3"));
deviationIds = new JSONArray(ImmutableSet.of("4", "5", "6"));
Assert.assertFalse(anyMatch(deviation, deviationIds));
}
}
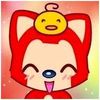
TA贡献1864条经验 获得超6个赞
任何匹配返回 true 的要求都可以重新表述为非空交集 return true。将JSONArrayinto转换Collection为很容易做到这一点,例如像这样
public class JsonArrayIntersectTest {
private boolean anyMatch(JSONArray first, JSONArray second) throws JSONException {
Set<Object> firstAsSet = convertJSONArrayToSet(first);
Set<Object> secondIdsAsSet = convertJSONArrayToSet(second);
//Set<Object> intersection = Sets.intersection(firstAsSet, secondIdsAsSet); // use this if you have Guava
Set<Object> intersection = firstAsSet.stream()
.distinct()
.filter(secondIdsAsSet::contains)
.collect(Collectors.toSet());
return !intersection.isEmpty();
}
Set<Object> convertJSONArrayToSet(JSONArray input) throws JSONException {
Set<Object> retVal = new HashSet<>();
for (int i = 0; i < input.length(); i++) {
retVal.add(input.get(i));
}
return retVal;
}
@Test
public void testCompareArrays() throws JSONException {
JSONArray deviation = new JSONArray(ImmutableSet.of("1", "2", "3"));
JSONArray deviationIds = new JSONArray(ImmutableSet.of("3", "4", "5"));
Assert.assertTrue(anyMatch(deviation, deviationIds));
deviation = new JSONArray(ImmutableSet.of("1", "2", "3"));
deviationIds = new JSONArray(ImmutableSet.of("4", "5", "6"));
Assert.assertFalse(anyMatch(deviation, deviationIds));
}
}
但是,与直接使用 API 的初始版本相比,代码要多得多JSONArray。它还会产生比直接 API 版本更多的迭代JSONArray,这可能是也可能不是问题。然而,总的来说,我认为在传输类型上实现领域逻辑并不是一个好的做法,例如JSONArray并且总是会转换成领域模型。
另请注意,生产就绪代码还会null对参数进行检查。
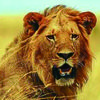
TA贡献1828条经验 获得超4个赞
您可以使用 XStream 来处理 JSON 映射
或者根据您检索字符串的逻辑,您可以比较为
JSONCompareResult result = JSONCompare.compareJSON(json1, json2, JSONCompareMode.STRICT); System.out.println(result.toString());
添加回答
举报