1 回答
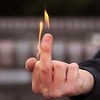
TA贡献1793条经验 获得超6个赞
到目前为止,我已经 [...]
byte[] data = new byte[bytesCount];
不幸的是,这种方法只有在您的位偏移量是 8 的倍数时才有效。在所有其他情况下,您必须划分要复制的每个字节。下图说明了如何划分每个字节以及将划分的部分放在哪里。
MSB = 最高有效位
LSB = 最低有效位
由于有很多极端情况,实现上述算法有点棘手。以下实现通过了您的所有测试和我的所有测试。我使用了许多变量来为所有计算赋予有意义的名称,希望它更容易理解。您可以通过消除其中一些变量并就地计算一些值来缩短实施时间。
我冒昧地将您的功能重命名toBytes为bitSubstring. 对于已经将字节作为输入的方法,以前的名称toBytes似乎有点不合时宜。
public static byte[] bitSubstring(int byteOffset, int bitOffset,
int lengthInBits, byte... source) {
return bitSubstring(8 * byteOffset + bitOffset, lengthInBits, source);
}
public static byte[] bitSubstring(int startBit, int lengthInBits,
byte... source) {
assert startBit >= 0 && startBit < 8 * source.length;
assert lengthInBits >= 0 && startBit + lengthInBits <= 8 * source.length;
int lengthInBytes = (int) Math.ceil(lengthInBits / 8.0);
byte[] target = new byte[lengthInBytes];
int startByte = startBit / 8;
int endBitExclusive = startBit + lengthInBits;
int endByteExclusive = (int) Math.ceil(endBitExclusive / 8.0);
int sourceBytesToRead = endByteExclusive - startByte;
int lowerPartSize = 8 * endByteExclusive - endBitExclusive;
int shiftLowerUp = (8 - lowerPartSize);
int shiftUpperDown = lowerPartSize;
int lastSrc = 0;
if (sourceBytesToRead > lengthInBytes) {
lastSrc = source[startByte] & 0xFF;
startByte++;
}
for (int targetByte = 0; targetByte < target.length; ++targetByte) {
int curSrc = source[startByte + targetByte] & 0xFF;
target[targetByte] |= (lastSrc << shiftLowerUp)
| (curSrc >>> shiftUpperDown);
lastSrc = curSrc;
}
int overhang = 8 * lengthInBytes - lengthInBits;
if (overhang > 0) {
target[0] &= 0xFF >>> overhang;
}
return target;
}
上面的算法应该相当快。但是,如果您只对实现大小和可读性感兴趣,那么逐位复制的方法会更好。
public static byte[] bitSubstringSlow(int startBitSource, int lengthInBits,
byte... source) {
byte[] target = new byte[(int) Math.ceil(lengthInBits / 8.0)];
int startBitTarget = (8 - lengthInBits % 8) % 8;
for (int i = 0; i < lengthInBits; ++i) {
setBit(target, startBitTarget + i, getBit(source, startBitSource + i));
}
return target;
}
public static int getBit(byte[] source, int bitIdx) {
return (source[bitIdx / 8] >>> (7 - bitIdx % 8)) & 1;
}
public static void setBit(byte[] target, int bitIdx, int bitValue) {
int block = bitIdx / 8;
int shift = 7 - bitIdx % 8;
target[block] &= ~(1 << shift);
target[block] |= bitValue << shift;
}
......或更少可重复使用但更短:
public static byte[] bitSubstringSlow2(int startBitSource, int lengthInBits,
byte... source) {
byte[] target = new byte[(int) Math.ceil(lengthInBits / 8.0)];
int startBitTarget = (8 - lengthInBits % 8) % 8;
for (int i = 0; i < lengthInBits; ++i) {
int srcIdx = startBitSource + i;
int tgtIdx = startBitTarget + i;
target[tgtIdx / 8] |= ((source[srcIdx / 8] >>> (7 - srcIdx % 8)) & 1)
<< (7 - tgtIdx % 8);
}
return target;
}
添加回答
举报