1 回答
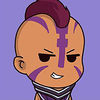
TA贡献1831条经验 获得超9个赞
const sleep = async (time) => {
return new Promise(resolve => setTimeout(resolve, time * 1000))
}
const sayHello = async () => {
await sleep(2)
console.log('Hello there')
}
sayHello()
这是解释:
使用setTimeout()which 是一种内置方法,可在指定的毫秒数后调用函数或计算表达式。 setTimeout()有两个参数,第一个是回调函数,第二个是毫秒数。1 秒 = 1000 毫秒所以 2 秒 = 2000 毫秒等等
function promised (val) {
// Create a new promise and resolve val after 2 seconds
return new Promise(resolve => setTimeout(() => resolve(val), 2000)) //2000ms => 2 seconds
}
const createPromise = promised('wait for it...') // Pass in your message to promised function
createPromise
.then(val => console.log(val))
// Catch errors if any you don't need it here since we are always resolving the promise i just included it here so you know it's exist
.catch(err => console.log(err))
你应该总是使用 .catch 除非你 100% 确定承诺总是会解决
例子:
function greeting(name) {
return new Promise((resolve, reject) => setTimeout(() => {
try {
if (name.length <= 2) {
throw new Error('Name must be more than two characters')
}
} catch (msg) {
reject(msg)
}
resolve(`Hello ${name}`)
}, 2000))
}
greeting('ab')
.then(res => console.log(res))
.catch(err => console.log(err)) // Error: Name must be more than two characters
greeting('John')
.then(res => console.log(res)) // Hello John
.catch(err => console.log(err))
使用异步,等待:
const greetSomeone = async (name) => {
try {
// Note that you can't use await outside an async function
const msg = await greeting(name)
console.log(msg)
} catch (err) {
console.log(err)
}
}
greetSomeone('ac') // Error: Name must be more than two characters
greetSomeone('Michael') // Hello Michael
添加回答
举报