3 回答
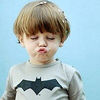
TA贡献1942条经验 获得超3个赞
var region = document.getElementById('region');
var town = document.getElementById('town');
var hotel = document.getElementById('hotel');
var json = {"regions":{"region1":{"Town1_1":["hotel1_1_1","hotel1_1_2"],"Town1_2":["hotel1_2_1","hotel1_2_2"],"Town1_3":["hotel1_3_1","hotel1_3_2"],"Town1_4":["hotel1_4_1","hotel1_4_2"]},"region2":{"Town2_1":["hotel2_1_1","hotel2_1_2"],"Town2_2":["hotel2_2_1","hotel2_2_2"],"Town2_3":["hotel2_3_1","hotel2_3_2"],"Town2_4":["hotel2_4_1","hotel2_4_2"],"Town2_5":["hotel2_5_1","hotel2_5_2"]},"region3":{"Town3_1":["hotel3_1_1","hotel3_1_2"],"Town3_2":["hotel3_2_1","hotel3_2_2"],"Town3_3":["hotel3_3_1","hotel3_3_2"],"Town3_4":["hotel3_4_1","hotel3_4_2"],"Town3_5":["hotel3_5_1","hotel3_5_2"],"Town3_6":["hotel3_6_1","hotel3_6_2"]}}};
region.innerHTML="";
var regions=Object.keys(json.regions);
regions.forEach(
regionkey=>{
var option=document.createElement("option");
option.textContent=regionkey;
region.append(option);
}
);
changetown();
changehotel();
function changetown() {
var selectedregion=region[region.selectedIndex].textContent;
town.innerHTML="";
var towns=Object.keys(json.regions[selectedregion]);
towns.forEach(
townkey=>{
var option=document.createElement("option");
option.textContent=townkey;
town.append(option);
}
);
changehotel();
}
function changehotel() {
var selectedregion=region[region.selectedIndex].textContent;
var selectedtown=town[town.selectedIndex].textContent;
hotel.innerHTML="";
var hotels=json.regions[selectedregion][selectedtown];
hotels.forEach(
hotelvalue=>{
var option=document.createElement("option");
option.textContent=hotelvalue;
hotel.append(option);
}
);
}
function dosomething() {
if(
(region.selectedIndex!=-1) &&
(town.selectedIndex!=-1) &&
(hotel.selectedIndex!=-1)
) {
var selectedregion=region[region.selectedIndex].textContent;
var selectedtown=town[town.selectedIndex].textContent;
var selectedhotel=hotel[hotel.selectedIndex].textContent;
console.log("Final: ", selectedregion, selectedtown, selectedhotel);
}
}
<select id="region" onchange="changetown()" ></select>
<select id="town" onchange="changehotel()"></select>
<select id="hotel" ></select>
<button id="go" onclick ="dosomething()">Go!</button>
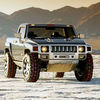
TA贡献1784条经验 获得超2个赞
这应该这样做:
const data = {regions:{region1:{
'Town1_1':['hotel1_1_1', 'hotel1_1_2'],'Town1_2':['hotel1_2_1', 'hotel1_2_2'],'Town1_3':['hotel1_3_1', 'hotel1_3_2'],'Town1_4':['hotel1_4_1', 'hotel1_4_2'],}, 'region2':{
'Town2_1':['hotel2_1_1', 'hotel2_1_2'],'Town2_2':['hotel2_2_1', 'hotel2_2_2'],'Town2_3':['hotel2_3_1', 'hotel2_3_2'],'Town2_4':['hotel2_4_1', 'hotel2_4_2'],'Town2_5':['hotel2_5_1', 'hotel2_5_2'],}, 'region3':{
'Town3_1':['hotel3_1_1', 'hotel3_1_2'],'Town3_2':['hotel3_2_1', 'hotel3_2_2'],'Town3_3':['hotel3_3_1', 'hotel3_3_2'],'Town3_4':['hotel3_4_1', 'hotel3_4_2'],'Town3_5':['hotel3_5_1', 'hotel3_5_2'],'Town3_6':['hotel3_6_1', 'hotel3_6_2'],}
}};
const mkopts=arr=>arr.map(r=>`<option>${r}</option>`).join(""),
sall= [...document.querySelectorAll('select')];
sall.forEach((sel,i)=>{
if (!i) sel.innerHTML=mkopts(Object.keys(data.regions)); // initially fill first select with options
if (i<2) { // change options of following select whenever one of the first two changes
sel.onchange=()=>{ let nsel=sall[i+1];
sall[2].innerHTML=""; // clear last select box options first
nsel.innerHTML=mkopts( // depending on situation (--> i ) get different options:
i ? Object.values(data.regions[sall[0].value][sall[1].value])
: Object.keys(data.regions[sall[0].value]) );
!i && nsel.onchange();
}
}
});
sall[0].onchange()
<select id='region'></select>
<select id='town'></select>
<select id='hotel'></select>
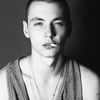
TA贡献1818条经验 获得超11个赞
更通用的解决方案怎么样?
var json = {'regions': {'region1': {'Town1_1': ['hotel1_1_1', 'hotel1_1_2'],'Town1_2': ['hotel1_2_1', 'hotel1_2_2'],'Town1_3': ['hotel1_3_1', 'hotel1_3_2'],'Town1_4': ['hotel1_4_1', 'hotel1_4_2'],},'region2': {'Town2_1': ['hotel2_1_1', 'hotel2_1_2'],'Town2_2': ['hotel2_2_1', 'hotel2_2_2'],'Town2_3': ['hotel2_3_1', 'hotel2_3_2'],'Town2_4': ['hotel2_4_1', 'hotel2_4_2'],'Town2_5': ['hotel2_5_1', 'hotel2_5_2'],},'region3': {'Town3_1': ['hotel3_1_1', 'hotel3_1_2'],'Town3_2': ['hotel3_2_1', 'hotel3_2_2'],'Town3_3': ['hotel3_3_1', 'hotel3_3_2'],'Town3_4': ['hotel3_4_1', 'hotel3_4_2'],'Town3_5': ['hotel3_5_1', 'hotel3_5_2'],'Town3_6': ['hotel3_6_1', 'hotel3_6_2'],},},
'heirarchy': ['region', 'town', 'hotel']};
function updateSelects(el) {
const heirarchy = json.heirarchy;
let selection = json.regions;
let level = json.heirarchy.indexOf(el);
for(let l=0;l<=level;l++) {
let text = document.getElementById(json.heirarchy[l]);
text = text.options[text.selectedIndex].text;
selection = selection[text]; // iterate selected levels
}
level++;
el = document.getElementById(heirarchy[level]);
for(var i=level;i<heirarchy.length;i++) {
clearSelect(document.getElementById(heirarchy[i]));
}
for (let i in selection) {
if (selection.constructor === Array) i = selection[i];
var opt1 = document.createElement('option');
opt1.innerText = i;
el.appendChild(opt1);
}
}
function clearSelect(el) {
while (el.options.length) el.removeChild(el.options[0]);
}
updateSelects();
<select id='region' onchange="updateSelects(this.id)"></select>
<select id='town' onchange="updateSelects(this.id)"></select>
<select id='hotel'></select>
添加回答
举报